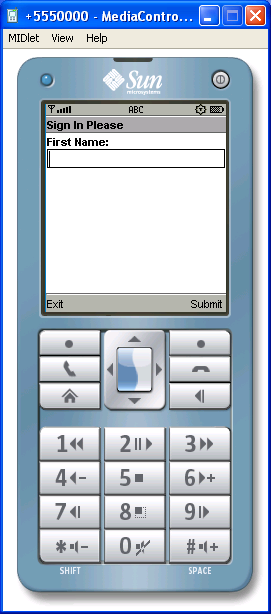
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.TextField;
import javax.microedition.midlet.MIDlet;
public class J2METextFieldCapture extends MIDlet implements CommandListener {
private Display display;
private Form form = new Form("Sign In Please");
private Command submit = new Command("Submit", Command.SCREEN, 1);
private Command exit = new Command("Exit", Command.EXIT, 1);
private TextField textfield = new TextField("First Name:", "", 30, TextField.ANY);
public J2METextFieldCapture() {
display = Display.getDisplay(this);
form.addCommand(exit);
form.addCommand(submit);
form.append(textfield);
form.setCommandListener(this);
}
public void startApp() {
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command command, Displayable displayable) {
if (command == submit) {
textfield.setString("Hello, " + textfield.getString());
form.removeCommand(submit);
} else if (command == exit) {
destroyApp(false);
notifyDestroyed();
}
}
}