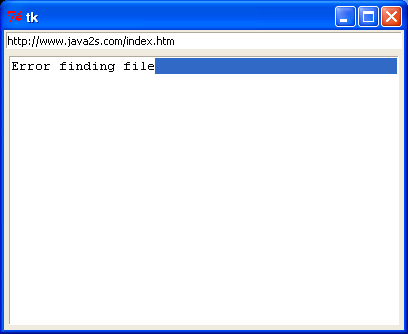
from Tkinter import *
import Pmw
import urllib
import urlparse
class WebBrowser( Frame ):
def __init__( self ):
Frame.__init__( self )
Pmw.initialise()
self.pack( expand = YES, fill = BOTH )
self.master.geometry( "400x300" )
self.address = Entry( self )
self.address.pack( fill = X, padx = 2, pady = 2 )
self.address.bind( "<Return>", self.getPage )
self.contents = Pmw.ScrolledText( self,text_state = DISABLED )
self.contents.pack( expand = YES, fill = BOTH, padx = 5,pady = 5 )
def getPage( self, event ):
myURL = event.widget.get()
components = urlparse.urlparse( myURL )
self.contents.text_state = NORMAL
if components[ 0 ] == "":
myURL = "http://" + myURL
try:
tempFile = urllib.urlopen( myURL )
self.contents.settext( tempFile.read() )
tempFile.close()
except IOError:
self.contents.settext( "Error finding file" )
self.contents.text_state = DISABLED
WebBrowser().mainloop()
18.3.Browser |
| 18.3.1. | Displays the contents of a file from a Web server in a browser. | 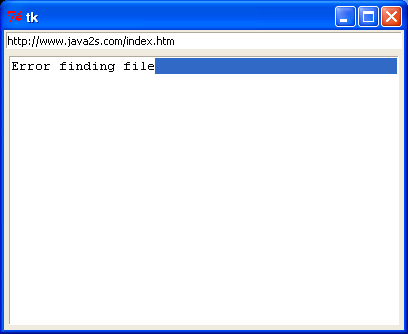 |