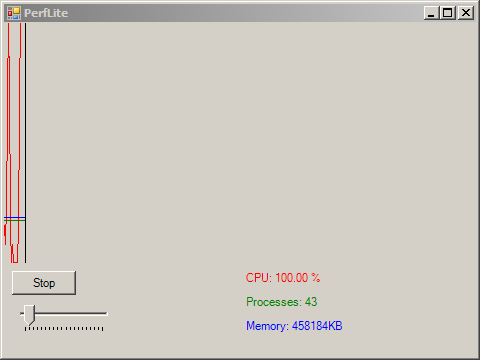
'Sams Teach Yourself Visual Basic .NET in 21 Days
'By Lowell Mauer
'Published 2001
'Sams Publishing
'ISBN 0672322714
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Drawing.Drawing2D
public class CharCPUMemory
public Shared Sub Main
Application.Run(New frmMain)
End Sub
End class
Public Class frmMain
Inherits System.Windows.Forms.Form
Dim m_sngX As Single
Dim m_sngY As Single
Dim m_sngCPUY As Single
Dim m_sngProcsY As Single
Dim m_sngMemY As Single
Dim penCPU As New System.Drawing.Pen(Color.Red)
Dim penProcs As New System.Drawing.Pen(Color.Green)
Dim penMem As New System.Drawing.Pen(Color.Blue)
Dim penFore As New System.Drawing.Pen(SystemColors.WindowText)
Dim penBack As New System.Drawing.Pen(SystemColors.Control)
Dim MachineName As String = System.Windows.Forms.SystemInformation.ComputerName
Const INCREMENT As Single = 1
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents pnlSweep As System.Windows.Forms.Panel
Friend WithEvents cmdSweep As System.Windows.Forms.Button
Friend WithEvents trkSpeed As System.Windows.Forms.TrackBar
Friend WithEvents lblCPU As System.Windows.Forms.Label
Friend WithEvents lblProc As System.Windows.Forms.Label
Friend WithEvents lblMem As System.Windows.Forms.Label
Friend WithEvents tmrClock As System.Windows.Forms.Timer
#Region "Declaration of Performance counters"
Friend WithEvents prfCPU As System.Diagnostics.PerformanceCounter
Friend WithEvents prfProcs As System.Diagnostics.PerformanceCounter
Friend WithEvents prfMem As System.Diagnostics.PerformanceCounter
#End Region
Friend WithEvents logApp As System.Diagnostics.EventLog
Private components As System.ComponentModel.IContainer
'Required by the Windows Form Designer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container()
Me.prfCPU = New System.Diagnostics.PerformanceCounter()
Me.pnlSweep = New System.Windows.Forms.Panel()
Me.tmrClock = New System.Windows.Forms.Timer(Me.components)
Me.trkSpeed = New System.Windows.Forms.TrackBar()
Me.lblProc = New System.Windows.Forms.Label()
Me.prfProcs = New System.Diagnostics.PerformanceCounter()
Me.lblMem = New System.Windows.Forms.Label()
Me.prfMem = New System.Diagnostics.PerformanceCounter()
Me.lblCPU = New System.Windows.Forms.Label()
Me.cmdSweep = New System.Windows.Forms.Button()
Me.logApp = New System.Diagnostics.EventLog()
CType(Me.prfCPU, System.ComponentModel.ISupportInitialize).BeginInit()
CType(Me.trkSpeed, System.ComponentModel.ISupportInitialize).BeginInit()
CType(Me.prfProcs, System.ComponentModel.ISupportInitialize).BeginInit()
CType(Me.prfMem, System.ComponentModel.ISupportInitialize).BeginInit()
CType(Me.logApp, System.ComponentModel.ISupportInitialize).BeginInit()
Me.SuspendLayout()
'***Initializing Performance Counters"
'
'prfCPU
'
Me.prfCPU.CategoryName = "Processor"
Me.prfCPU.CounterName = "% Processor Time"
Me.prfCPU.InstanceName = "_Total"
Me.prfCPU.MachineName = MachineName
'
'prfProcs
'
Me.prfProcs.CategoryName = "System"
Me.prfProcs.CounterName = "Processes"
Me.prfProcs.MachineName = MachineName
'
'prfMem
'
Me.prfMem.CategoryName = "Memory"
Me.prfMem.CounterName = "Committed Bytes"
Me.prfMem.MachineName = MachineName
'
'pnlSweep
'
Me.pnlSweep.Dock = System.Windows.Forms.DockStyle.Top
Me.pnlSweep.Name = "pnlSweep"
Me.pnlSweep.Size = New System.Drawing.Size(472, 240)
Me.pnlSweep.TabIndex = 0
'
'tmrClock
'
Me.tmrClock.Interval = 1000
'
'trkSpeed
'
Me.trkSpeed.Location = New System.Drawing.Point(8, 280)
Me.trkSpeed.Maximum = 20
Me.trkSpeed.Minimum = 1
Me.trkSpeed.Name = "trkSpeed"
Me.trkSpeed.TabIndex = 2
Me.trkSpeed.Value = 10
'
'lblProc
'
Me.lblProc.AutoSize = True
Me.lblProc.ForeColor = System.Drawing.Color.Green
Me.lblProc.Location = New System.Drawing.Point(240, 272)
Me.lblProc.Name = "lblProc"
Me.lblProc.Size = New System.Drawing.Size(60, 13)
Me.lblProc.TabIndex = 3
Me.lblProc.Text = "Processes:"
'
'lblMem
'
Me.lblMem.AutoSize = True
Me.lblMem.ForeColor = System.Drawing.Color.Blue
Me.lblMem.Location = New System.Drawing.Point(240, 296)
Me.lblMem.Name = "lblMem"
Me.lblMem.Size = New System.Drawing.Size(48, 13)
Me.lblMem.TabIndex = 3
Me.lblMem.Text = "Memory:"
'
'lblCPU
'
Me.lblCPU.AutoSize = True
Me.lblCPU.ForeColor = System.Drawing.Color.Red
Me.lblCPU.Location = New System.Drawing.Point(240, 248)
Me.lblCPU.Name = "lblCPU"
Me.lblCPU.Size = New System.Drawing.Size(31, 13)
Me.lblCPU.TabIndex = 3
Me.lblCPU.Text = "CPU:"
'
'cmdSweep
'
Me.cmdSweep.Location = New System.Drawing.Point(8, 248)
Me.cmdSweep.Name = "cmdSweep"
Me.cmdSweep.Size = New System.Drawing.Size(64, 24)
Me.cmdSweep.TabIndex = 1
Me.cmdSweep.Text = "&Start"
'
'logApp
'
Me.logApp.Log = "Application"
Me.logApp.MachineName = MachineName
Me.logApp.Source = "PerfLite"
Me.logApp.SynchronizingObject = Me
'
'frmMain
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(472, 333)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblCPU, Me.trkSpeed, Me.cmdSweep, Me.pnlSweep, Me.lblProc, Me.lblMem})
Me.Name = "frmMain"
Me.Text = "PerfLite"
CType(Me.prfCPU, System.ComponentModel.ISupportInitialize).EndInit()
CType(Me.trkSpeed, System.ComponentModel.ISupportInitialize).EndInit()
CType(Me.prfProcs, System.ComponentModel.ISupportInitialize).EndInit()
CType(Me.prfMem, System.ComponentModel.ISupportInitialize).EndInit()
CType(Me.logApp, System.ComponentModel.ISupportInitialize).EndInit()
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub tmrClock_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles tmrClock.Tick
Dim sngCPU As Single = prfCPU.NextValue() / 100
Dim sngProcs As Single = prfProcs.NextValue()
Dim sngMem As Single = prfMem.NextValue / 1024
'draw the sweep panel
DrawSweep(sngCPU, sngProcs, sngMem)
'update the labels
lblCPU.Text = "CPU: " & sngCPU.ToString("p")
lblProc.Text = "Processes: " & sngProcs
lblMem.Text = "Memory: " & sngMem.ToString("f0") & "KB"
End Sub
Private Sub DrawSweep(ByVal CPU As Single, _
ByVal Processes As Single, _
ByVal Memory As Single)
Dim oGrafix As Graphics = pnlSweep.CreateGraphics()
Dim sngHeight As Single = pnlSweep.Height
'for the points
Dim sngCPUY As Single
Dim sngProcsY As Single
Dim sngMemY As Single
'erase previous sweep line
oGrafix.DrawLine(penBack, m_sngX, 0, m_sngX, sngHeight)
'draw data points
sngCPUY = sngHeight - (CPU * sngHeight) - 1
oGrafix.DrawLine(penCPU, _
m_sngX - INCREMENT, m_sngCPUY, m_sngX, sngCPUY)
m_sngCPUY = sngCPUY
sngProcsY = sngHeight - Processes
oGrafix.DrawLine(penProcs, _
m_sngX - INCREMENT, m_sngProcsY, m_sngX, sngProcsY)
m_sngProcsY = sngProcsY
'the 10000 is for my machine, to get the memory to a nice value
' you may need to change it if the memory line
' isn't drawing correctly
sngMemY = sngHeight - (Memory / 10000)
oGrafix.DrawLine(penMem, _
m_sngX - INCREMENT, m_sngMemY, m_sngX, sngMemY)
m_sngMemY = sngMemY
'increment x
m_sngX += INCREMENT
If m_sngX > pnlSweep.Width Then
'reset back to start
m_sngX = 0
'and clear the drawing surface
oGrafix.Clear(SystemColors.Control)
End If
'draw new line
oGrafix.DrawLine(penFore, m_sngX, 0, m_sngX, sngHeight)
End Sub
Private Sub cmdSweep_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdSweep.Click
'Toggle the button text and timer (on or off)
If cmdSweep.Text = "&Start" Then
cmdSweep.Text = "&Stop"
tmrClock.Enabled = True
Else
cmdSweep.Text = "&Start"
tmrClock.Enabled = False
End If
End Sub
Private Sub trkSpeed_Scroll(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles trkSpeed.Scroll
Dim iValue As Integer
iValue = CInt(trkSpeed.Value)
'set the timer interval to the selected time
tmrClock.Interval = iValue * 100 'ms
End Sub
End Class