<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Loaded="OnLoad" xmlns:ds="clr-namespace:WpfApplication1">
<Window.Resources>
<ObjectDataProvider x:Key="EmployeeInfoDataSource" ObjectType="{x:Type ds:myEmployees}"/>
</Window.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="50"/>
<RowDefinition/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" FontSize="14" HorizontalAlignment="Center">
ListView created with XAML
</TextBlock>
<StackPanel Grid.Row="1" Grid.Column="0" HorizontalAlignment="Center">
<ListView ItemsSource="{Binding Source={StaticResource EmployeeInfoDataSource}}">
<ListView.View>
<GridView AllowsColumnReorder="true" ColumnHeaderToolTip="Employee Information">
<GridViewColumn DisplayMemberBinding= "{Binding Path=FirstName}" Header="First Name" Width="100"/>
<GridViewColumn DisplayMemberBinding="{Binding Path=EmployeeNumber}" Header="Employee No." Width="100"/>
</GridView>
</ListView.View>
</ListView>
</StackPanel>
<StackPanel Grid.Row="1" Grid.Column="1" Name="myStackPanel" HorizontalAlignment="Center">
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Imports System.Collections.ObjectModel
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Private Sub OnLoad(sender As Object, e As RoutedEventArgs)
Dim myListView As New ListView()
Dim myGridView As New GridView()
myGridView.AllowsColumnReorder = True
myGridView.ColumnHeaderToolTip = "Employee Information"
Dim gvc1 As New GridViewColumn()
gvc1.DisplayMemberBinding = New Binding("FirstName")
gvc1.Header = "FirstName"
gvc1.Width = 100
myGridView.Columns.Add(gvc1)
Dim gvc3 As New GridViewColumn()
gvc3.DisplayMemberBinding = New Binding("EmployeeNumber")
gvc3.Header = "Employee No."
gvc3.Width = 100
myGridView.Columns.Add(gvc3)
myListView.ItemsSource = New myEmployees()
myListView.View = myGridView
myStackPanel.Children.Add(myListView)
End Sub
End Class
Public Class EmployeeInfo
Private _firstName As String
Private _employeeNumber As String
Public Property FirstName() As String
Get
Return _firstName
End Get
Set
_firstName = value
End Set
End Property
Public Property EmployeeNumber() As String
Get
Return _employeeNumber
End Get
Set
_employeeNumber = value
End Set
End Property
Public Sub New(firstname As String, empnumber As String)
_firstName = firstname
_employeeNumber = empnumber
End Sub
End Class
Public Class myEmployees
Inherits ObservableCollection(Of EmployeeInfo)
Public Sub New()
Add(New EmployeeInfo("A", "1"))
Add(New EmployeeInfo("B", "9"))
Add(New EmployeeInfo("C", "2"))
Add(New EmployeeInfo("D", "4"))
End Sub
End Class
End Namespace
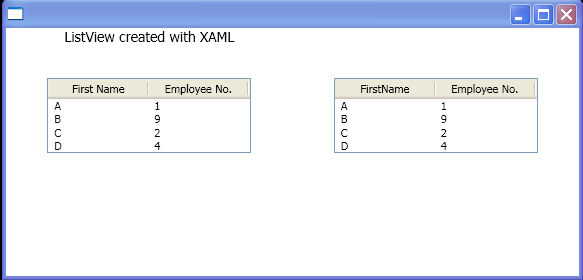