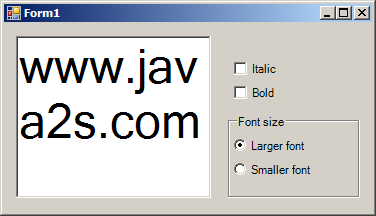
Imports System.Windows.Forms
public class RadioButtonControlFont
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Public mysize As Integer
Public mybold As Boolean
Public myItalic As Boolean
Private Sub RadioButton1_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs)
CheckBox1.Checked = 1
End Sub
Private Sub CheckBox1_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles CheckBox1.CheckedChanged
myItalic = Not myItalic
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Regular)
If myItalic And mybold Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Italic)
ElseIf myItalic Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Italic)
ElseIf mybold Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Bold)
End If
End Sub
Private Sub GroupBox1_Enter(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles GroupBox1.Enter
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
mybold = False
myItalic = False
mysize = 25
End Sub
Private Sub RadioButton1_CheckedChanged_1(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton1.CheckedChanged
mysize = 36
TextBox1.Font = New System.Drawing.Font("", mysize)
End Sub
Private Sub RadioButton2_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton2.CheckedChanged
mysize = 25
TextBox1.Font = New System.Drawing.Font("", mysize)
End Sub
Private Sub CheckBox2_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles CheckBox2.CheckedChanged
mybold = Not mybold
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Regular)
If myItalic And mybold Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Bold)
ElseIf myItalic Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Italic)
ElseIf mybold Then
TextBox1.Font = New System.Drawing.Font("", mysize, Drawing.FontStyle.Bold)
End If
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.CheckBox1 = New System.Windows.Forms.CheckBox
Me.CheckBox2 = New System.Windows.Forms.CheckBox
Me.GroupBox1 = New System.Windows.Forms.GroupBox
Me.RadioButton1 = New System.Windows.Forms.RadioButton
Me.RadioButton2 = New System.Windows.Forms.RadioButton
Me.TextBox1 = New System.Windows.Forms.TextBox
Me.GroupBox1.SuspendLayout()
Me.SuspendLayout()
'
'CheckBox1
'
Me.CheckBox1.AutoSize = True
Me.CheckBox1.Location = New System.Drawing.Point(230, 34)
Me.CheckBox1.Name = "CheckBox1"
Me.CheckBox1.Size = New System.Drawing.Size(48, 16)
Me.CheckBox1.TabIndex = 1
Me.CheckBox1.Text = "Italic"
Me.CheckBox1.UseVisualStyleBackColor = True
'
'CheckBox2
'
Me.CheckBox2.AutoSize = True
Me.CheckBox2.Location = New System.Drawing.Point(230, 56)
Me.CheckBox2.Name = "CheckBox2"
Me.CheckBox2.Size = New System.Drawing.Size(48, 16)
Me.CheckBox2.TabIndex = 2
Me.CheckBox2.Text = "Bold"
Me.CheckBox2.UseVisualStyleBackColor = True
'
'GroupBox1
'
Me.GroupBox1.Controls.Add(Me.RadioButton2)
Me.GroupBox1.Controls.Add(Me.RadioButton1)
Me.GroupBox1.Location = New System.Drawing.Point(224, 85)
Me.GroupBox1.Name = "GroupBox1"
Me.GroupBox1.Size = New System.Drawing.Size(132, 77)
Me.GroupBox1.TabIndex = 3
Me.GroupBox1.TabStop = False
Me.GroupBox1.Text = "Font size"
'
'RadioButton1
'
Me.RadioButton1.AutoSize = True
Me.RadioButton1.Location = New System.Drawing.Point(6, 20)
Me.RadioButton1.Name = "RadioButton1"
Me.RadioButton1.Size = New System.Drawing.Size(59, 16)
Me.RadioButton1.TabIndex = 1
Me.RadioButton1.TabStop = True
Me.RadioButton1.Text = "Larger font"
Me.RadioButton1.UseVisualStyleBackColor = True
'
'RadioButton2
'
Me.RadioButton2.AutoSize = True
Me.RadioButton2.Location = New System.Drawing.Point(6, 42)
Me.RadioButton2.Name = "RadioButton2"
Me.RadioButton2.Size = New System.Drawing.Size(59, 16)
Me.RadioButton2.TabIndex = 2
Me.RadioButton2.TabStop = True
Me.RadioButton2.Text = "Smaller font"
Me.RadioButton2.UseVisualStyleBackColor = True
'
'TextBox1
'
Me.TextBox1.Location = New System.Drawing.Point(12, 12)
Me.TextBox1.Multiline = True
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(195, 150)
Me.TextBox1.TabIndex = 4
Me.TextBox1.Text = "www.java2s.com"
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 12.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(368, 174)
Me.Controls.Add(Me.TextBox1)
Me.Controls.Add(Me.GroupBox1)
Me.Controls.Add(Me.CheckBox2)
Me.Controls.Add(Me.CheckBox1)
Me.Name = "Form1"
Me.Text = "Form1"
Me.GroupBox1.ResumeLayout(False)
Me.GroupBox1.PerformLayout()
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents CheckBox1 As System.Windows.Forms.CheckBox
Friend WithEvents CheckBox2 As System.Windows.Forms.CheckBox
Friend WithEvents GroupBox1 As System.Windows.Forms.GroupBox
Friend WithEvents RadioButton2 As System.Windows.Forms.RadioButton
Friend WithEvents RadioButton1 As System.Windows.Forms.RadioButton
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
End Class