Gradient Brushes
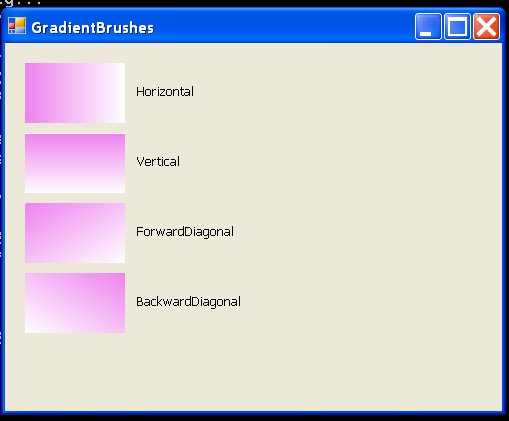
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace GDI_Basics
{
/// <summary>
/// Summary description for GradientBrushes.
/// </summary>
public class GradientBrushes : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public GradientBrushes()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// GradientBrushes
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(388, 298);
this.Name = "GradientBrushes";
this.Text = "GradientBrushes";
this.Resize += new System.EventHandler(this.GradientBrushes_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.GradientBrushes_Paint);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new GradientBrushes());
}
private void GradientBrushes_Resize(object sender, System.EventArgs e)
{
this.Invalidate();
}
private void GradientBrushes_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
ArrayList stylesShown = new ArrayList();
LinearGradientBrush myBrush;
int y = 20;
int x = 20;
foreach (LinearGradientMode gradientStyle in System.Enum.GetValues(typeof(LinearGradientMode)))
{
myBrush = new LinearGradientBrush(new Rectangle(x, y, 100, 60), Color.Violet, Color.White, gradientStyle);
e.Graphics.FillRectangle(myBrush, x, y, 100, 60);
e.Graphics.DrawString(gradientStyle.ToString(), new Font("Tahoma", 8), Brushes.Black, 110 + x, y + 20);
y += 70;
}
}
}
}
Related examples in the same category