Path Gradient Brush from Graphics Path
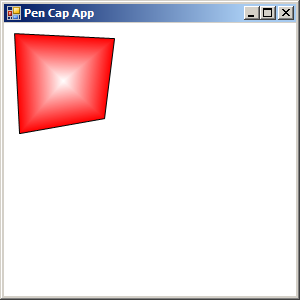
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class Form1 : System.Windows.Forms.Form
{
public Form1()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "Pen Cap App";
this.Resize += new System.EventHandler(this.Form1_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
GraphicsPath gp = new GraphicsPath();
gp.AddLine(10, 10, 110, 15);
gp.AddLine(110, 15, 100, 95);
gp.AddLine(100, 95, 15, 110);
gp.CloseFigure();
g.FillRectangle(Brushes.White, this.ClientRectangle);
g.SmoothingMode = SmoothingMode.AntiAlias;
PathGradientBrush pgb = new PathGradientBrush(gp);
pgb.CenterColor = Color.White;
pgb.SurroundColors = new Color[] { Color.Red };
g.FillPath(pgb, gp);
g.DrawPath(Pens.Black, gp);
pgb.Dispose();
gp.Dispose();
}
private void Form1_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}
Related examples in the same category