Path Gradient Demo
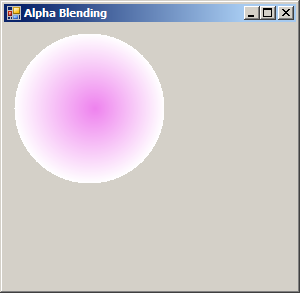
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
public class Form1 : Form
{
public Form1() {
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
GraphicsPath path = new GraphicsPath();
int size = 150;
path.AddEllipse(10, 10, size, size);
PathGradientBrush brush = new PathGradientBrush(path);
brush.WrapMode = WrapMode.Tile;
brush.SurroundColors = new Color[] { Color.White };
brush.CenterColor = Color.Violet;
e.Graphics.FillRectangle(brush, 10, 10, size, size);
path.Dispose();
brush.Dispose();
}
private void InitializeComponent()
{
this.SuspendLayout();
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "Form1";
this.Text = "Alpha Blending";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}
Related examples in the same category