Handle button messages
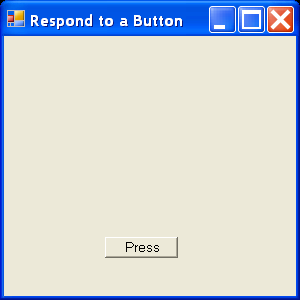
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Handle button messages.
using System;
using System.Windows.Forms;
using System.Drawing;
public class ButtonForm1 : Form {
Button MyButton = new Button();
public ButtonForm1() {
Text = "Respond to a Button";
MyButton = new Button();
MyButton.Text = "Press Here";
MyButton.Location = new Point(100, 200);
// Add button event handler to list.
MyButton.Click += new EventHandler(MyButtonClick);
Controls.Add(MyButton);
}
[STAThread]
public static void Main() {
ButtonForm1 skel = new ButtonForm1();
Application.Run(skel);
}
// Handler for MyButton.
protected void MyButtonClick(object who, EventArgs e) {
if(MyButton.Top == 200)
MyButton.Location = new Point(10, 10);
else
MyButton.Location = new Point(100, 200);
}
}
Related examples in the same category