Canvas for processing key code and commands
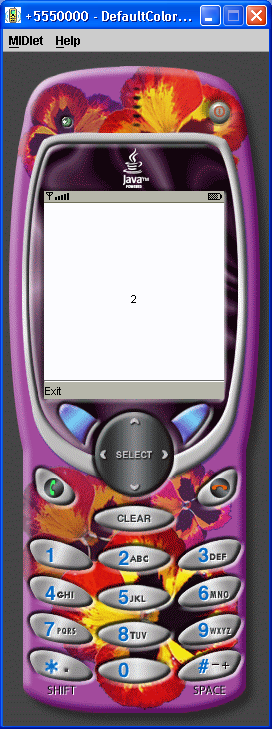
/*--------------------------------------------------
* KeyCodes.java
*
* Canvas for processing key code and commands
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class KeyCodes extends MIDlet
{
private Display display; // The display
private KeyCodeCanvas canvas; // Canvas
public KeyCodes()
{
display = Display.getDisplay(this);
canvas = new KeyCodeCanvas(this);
}
protected void startApp()
{
display.setCurrent( canvas );
}
protected void pauseApp()
{ }
protected void destroyApp( boolean unconditional )
{ }
public void exitMIDlet()
{
destroyApp(true);
notifyDestroyed();
}
}
/*--------------------------------------------------
* Class KeyCodeCanvas
*
* Key codes and commands for event handling
*-------------------------------------------------*/
class KeyCodeCanvas extends Canvas implements CommandListener
{
private Command cmExit; // Exit midlet
private String keyText = null; // Key code text
private KeyCodes midlet;
/*--------------------------------------------------
* Constructor
*-------------------------------------------------*/
public KeyCodeCanvas(KeyCodes midlet)
{
this.midlet = midlet;
// Create exit command & listen for events
cmExit = new Command("Exit", Command.EXIT, 1);
addCommand(cmExit);
setCommandListener(this);
}
/*--------------------------------------------------
* Paint the text representing the key code
*-------------------------------------------------*/
protected void paint(Graphics g)
{
// Clear the background (to white)
g.setColor(255, 255, 255);
g.fillRect(0, 0, getWidth(), getHeight());
// Set color and draw text
if (keyText != null)
{
// Draw with black pen
g.setColor(0, 0, 0);
// Close to the center
g.drawString(keyText, getWidth()/2, getHeight()/2, Graphics.TOP | Graphics.HCENTER);
}
}
/*--------------------------------------------------
* Command event handling
*-------------------------------------------------*/
public void commandAction(Command c, Displayable d)
{
if (c == cmExit)
midlet.exitMIDlet();
}
/*--------------------------------------------------
* Key code event handling
*-------------------------------------------------*/
protected void keyPressed(int keyCode)
{
keyText = getKeyName(keyCode);
repaint();
}
}
Related examples in the same category