Key MIDlet
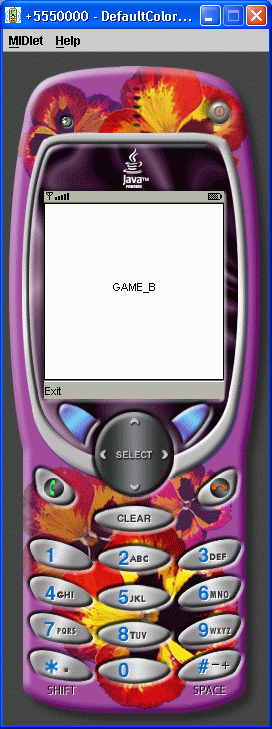
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Font;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class KeyMIDlet extends MIDlet {
public void startApp() {
Displayable d = new KeyCanvas();
d.addCommand(new Command("Exit", Command.EXIT, 0));
d.setCommandListener(new CommandListener() {
public void commandAction(Command c, Displayable s) {
notifyDestroyed();
}
});
Display.getDisplay(this).setCurrent(d);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
class KeyCanvas extends Canvas {
private Font mFont = Font.getFont(Font.FACE_PROPORTIONAL, Font.STYLE_PLAIN, Font.SIZE_MEDIUM);
private String mMessage = "[Press keys]";
public KeyCanvas() {
}
public void paint(Graphics g) {
int w = getWidth();
int h = getHeight();
g.setGrayScale(255);
g.fillRect(0, 0, w - 1, h - 1);
g.setGrayScale(0);
g.drawRect(0, 0, w - 1, h - 1);
g.setFont(mFont);
int x = w / 2;
int y = h / 2;
g.drawString(mMessage, x, y, Graphics.BASELINE | Graphics.HCENTER);
}
protected void keyPressed(int keyCode) {
int gameAction = getGameAction(keyCode);
switch (gameAction) {
case UP:
mMessage = "UP";
break;
case DOWN:
mMessage = "DOWN";
break;
case LEFT:
mMessage = "LEFT";
break;
case RIGHT:
mMessage = "RIGHT";
break;
case FIRE:
mMessage = "FIRE";
break;
case GAME_A:
mMessage = "GAME_A";
break;
case GAME_B:
mMessage = "GAME_B";
break;
case GAME_C:
mMessage = "GAME_C";
break;
case GAME_D:
mMessage = "GAME_D";
break;
default:
mMessage = "";
break;
}
repaint();
}
}
Related examples in the same category