Change Label Text
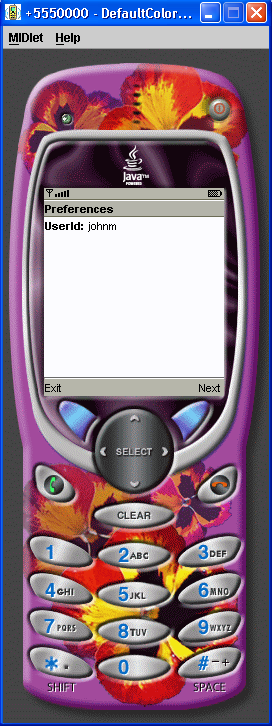
/*--------------------------------------------------
* ChangeLabelText.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class ChangeLabelText extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // The main form
private StringItem siUser; // The message
private Command cmNext; // Next label and message
private Command cmExit; // Command to exit the MIDlet
public ChangeLabelText()
{
display = Display.getDisplay(this);
// Create text message and commands
siUser = new StringItem("UserId: ", "johnm");
cmNext = new Command("Next", Command.SCREEN, 1);
cmExit = new Command("Exit", Command.EXIT, 1);
// Create Form, add Command & StringItem, listen for events
fmMain = new Form("Preferences");
fmMain.addCommand(cmExit);
fmMain.addCommand(cmNext);
fmMain.append(siUser);
fmMain.setCommandListener(this);
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmNext)
{
// This method is inherited from the Item class
siUser.setLabel("Account #: ");
// Change the text
siUser.setText("731");
// Remove the Next command
fmMain.removeCommand(cmNext);
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
}
Related examples in the same category