Read Display File
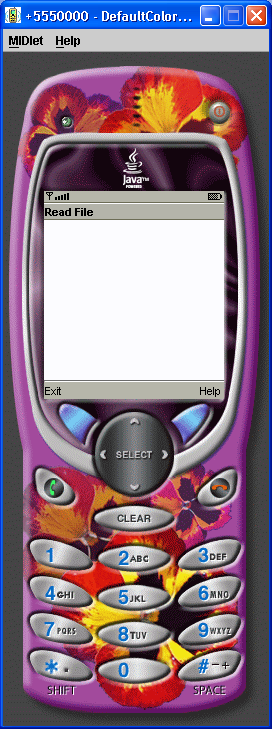
/*--------------------------------------------------
* ReadDisplayFile.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
import java.io.*;
public class ReadDisplayFile extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // Main form
private Command cmHelp; // Command to show a help file
private Command cmExit; // Command to exit the MIDlet
private Alert alHelp; // Alert to display help file text
public ReadDisplayFile()
{
display = Display.getDisplay(this);
cmHelp = new Command("Help", Command.SCREEN, 1);
cmExit = new Command("Exit", Command.EXIT, 1);
fmMain = new Form("Read File");
fmMain.addCommand(cmExit);
fmMain.addCommand(cmHelp);
fmMain.setCommandListener(this);
}
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmHelp)
{
String str;
// Access the resource and read its contents
if ((str = readHelpText()) != null)
{
// Create an Alert to display the help text
alHelp = new Alert("Help", str, null, null);
alHelp.setTimeout(Alert.FOREVER);
display.setCurrent(alHelp, fmMain);
}
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
private String readHelpText()
{
InputStream is = getClass().getResourceAsStream("help.txt");
try
{
StringBuffer sb = new StringBuffer();
int chr, i = 0;
// Read until the end of the stream
while ((chr = is.read()) != -1)
sb.append((char) chr);
return sb.toString();
}
catch (Exception e)
{
System.out.println("Unable to create stream");
}
return null;
}
}
Related examples in the same category