Display Stats
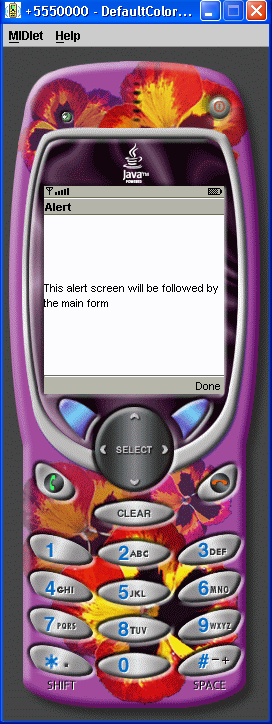
/*--------------------------------------------------
* DisplayStats.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class DisplayStats extends MIDlet implements CommandListener
{
private Display display; // Reference to Display object
private Form fmMain; // A Form
private Alert alTest; // An Alert
private Command cmExit; // A Command to exit the MIDlet
public DisplayStats()
{
display = Display.getDisplay(this);
cmExit = new Command("Exit", Command.SCREEN, 1);
fmMain = new Form("Welcome");
fmMain.addCommand(cmExit);
fmMain.setCommandListener(this);
System.out.println("Display " + (display.isColor() ? "does" : "does not") + " support Color");
System.out.println("Number of colors: " + display.numColors());
}
// Called by application manager to start the MIDlet.
public void startApp()
{
alTest = new Alert("Alert", "This alert screen will be followed by the main form", null, null);
alTest.setTimeout(Alert.FOREVER);
display.setCurrent(alTest, fmMain);
}
// We are about to be placed in the Paused state
public void pauseApp()
{
}
// We are about to enter the Destroyed state
public void destroyApp(boolean unconditional)
{
}
// Check to see if the Exit command was selected
public void commandAction(Command c, Displayable s)
{
if (c == cmExit)
{
destroyApp(true);
notifyDestroyed();
}
}
}
Related examples in the same category