Draw immutable image on a canvas
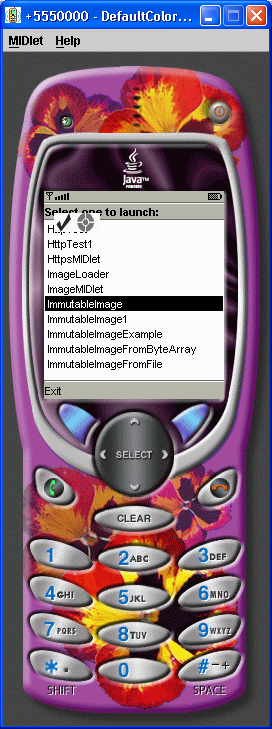
/*--------------------------------------------------
* ImmutableImage.java
*
* Draw immutable image on a canvas
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class ImmutableImage extends MIDlet
{
private Display display; // The display
private ImageCanvas canvas; // Canvas
public ImmutableImage()
{
display = Display.getDisplay(this);
canvas = new ImageCanvas(this);
}
protected void startApp()
{
display.setCurrent( canvas );
}
protected void pauseApp()
{ }
protected void destroyApp( boolean unconditional )
{ }
public void exitMIDlet()
{
destroyApp(true);
notifyDestroyed();
}
}
/*--------------------------------------------------
* Class ImageCanvas
*
* Draw immutable image
*-------------------------------------------------*/
class ImageCanvas extends Canvas implements CommandListener
{
private Command cmExit; // Exit midlet
private ImmutableImage midlet;
private Image im = null;
public ImageCanvas(ImmutableImage midlet)
{
this.midlet = midlet;
// Create exit command & listen for events
cmExit = new Command("Exit", Command.EXIT, 1);
addCommand(cmExit);
setCommandListener(this);
try
{
// Create immutable image
im = Image.createImage("/image_bw.png");
}
catch (java.io.IOException e)
{
System.err.println("Unable to locate or read .png file");
}
}
/*--------------------------------------------------
* Draw immutable image
*-------------------------------------------------*/
protected void paint(Graphics g)
{
if (im != null)
g.drawImage(im, 10, 10, Graphics.LEFT | Graphics.TOP);
}
public void commandAction(Command c, Displayable d)
{
if (c == cmExit)
midlet.exitMIDlet();
}
}
Related examples in the same category