MutableImage
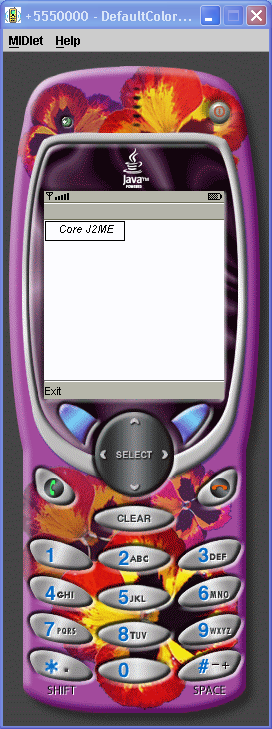
/*--------------------------------------------------
* MutableImage.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class MutableImage extends MIDlet implements CommandListener
{
private Display display; // Reference to display object
private Form fmMain; // Main form
private Command cmExit; // Command to exit the MIDlet
private static final String message = "Core J2ME";
public MutableImage()
{
display = Display.getDisplay(this);
// Create a mutable image and get graphics object for image
Image tmpImg = Image.createImage(80, 20);
Graphics graphics = tmpImg.getGraphics();
// Specify a font face, style and size
Font font = Font.getFont(Font.FACE_SYSTEM, Font.STYLE_ITALIC, Font.SIZE_MEDIUM);
graphics.setFont(font);
// Center the text in the image
graphics.drawString(message,
(tmpImg.getWidth() / 2) - (font.stringWidth(message) / 2), 0,
Graphics.TOP | Graphics.LEFT);
// Draw a rectangle around the image
graphics.drawRect(0,0, tmpImg.getWidth()-1, tmpImg.getHeight()-1);
cmExit = new Command("Exit", Command.EXIT, 1);
fmMain = new Form("");
fmMain.addCommand(cmExit);
fmMain.setCommandListener(this);
// Convert the image to immutable and add to the form
fmMain.append(Image.createImage(tmpImg));
display.setCurrent(fmMain);
}
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{
}
public void destroyApp(boolean unconditional)
{
}
public void commandAction(Command c, Displayable s)
{
if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
}
Related examples in the same category