Customized layout manager
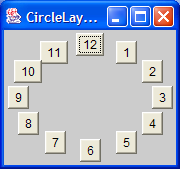
import java.awt.Button;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.Insets;
import java.awt.LayoutManager;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JFrame;
public class CircleLayoutTest extends JFrame {
public CircleLayoutTest() {
setTitle("CircleLayoutTest");
setSize(300, 300);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
getContentPane().setLayout(new CircleLayout());
getContentPane().add(new Button("3"));
getContentPane().add(new Button("4"));
getContentPane().add(new Button("5"));
getContentPane().add(new Button("6"));
getContentPane().add(new Button("7"));
getContentPane().add(new Button("8"));
getContentPane().add(new Button("9"));
getContentPane().add(new Button("10"));
getContentPane().add(new Button("11"));
getContentPane().add(new Button("12"));
getContentPane().add(new Button("1"));
getContentPane().add(new Button("2"));
}
public static void main(String[] args) {
JFrame f = new CircleLayoutTest();
f.show();
}
}
class CircleLayout implements LayoutManager {
private int minWidth = 0;
private int minHeight = 0;
private int preferredWidth = 0, preferredHeight = 0;
private boolean sizesSet = false;
private int maxComponentWidth = 0;
private int maxComponentHeight = 0;
public void addLayoutComponent(String name, Component comp) {
}
public void removeLayoutComponent(Component comp) {
}
public void setSizes(Container parent) {
if (sizesSet)
return;
int comCount = parent.getComponentCount();
for (int i = 0; i < comCount; i++) {
Component c = parent.getComponent(i);
if (c.isVisible()) {
Dimension size = c.getPreferredSize();
maxComponentWidth = Math.max(maxComponentWidth, size.width);
maxComponentHeight = Math.max(maxComponentWidth, size.height);
preferredHeight += size.height;
}
}
preferredHeight += maxComponentHeight;
preferredWidth = 2 * maxComponentWidth;
minHeight = preferredHeight;
minWidth = preferredWidth;
sizesSet = true;
}
public Dimension preferredLayoutSize(Container parent) {
Dimension dim = new Dimension(0, 0);
setSizes(parent);
Insets insets = parent.getInsets();
dim.width = preferredWidth + insets.left + insets.right;
dim.height = preferredHeight + insets.top + insets.bottom;
return dim;
}
public Dimension minimumLayoutSize(Container parent) {
return preferredLayoutSize(parent);
}
public void layoutContainer(Container parent) {
Insets insets = parent.getInsets();
int containerWidth = parent.getSize().width - insets.left
- insets.right;
int containerHeight = parent.getSize().height - insets.top
- insets.bottom;
int xradius = (containerWidth - maxComponentWidth) / 2;
int yradius = (containerHeight - maxComponentHeight) / 2;
setSizes(parent);
int centerX = insets.left + containerWidth / 2;
int centerY = insets.top + containerHeight / 2;
int comCount = parent.getComponentCount();
for (int i = 0; i < comCount; i++) {
Component c = parent.getComponent(i);
if (c.isVisible()) {
Dimension size = c.getPreferredSize();
double angle = 2 * Math.PI * i / comCount;
int x = centerX + (int) (Math.cos(angle) * xradius);
int y = centerY + (int) (Math.sin(angle) * yradius);
c.setBounds(x - size.width / 2, y - size.height / 2, size.width,
size.height);
}
}
}
}
Related examples in the same category