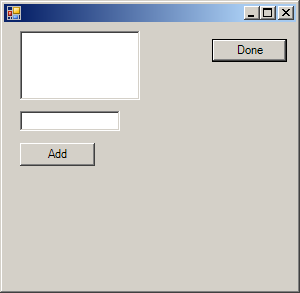
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class ListBoxContextMenu : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnDone;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Button btnAdd;
private System.Windows.Forms.ListBox listBox1;
private System.Windows.Forms.ContextMenu contextMenu1;
private System.Windows.Forms.MenuItem menuItem1;
private System.Windows.Forms.MenuItem menuItem2;
private System.Windows.Forms.MenuItem menuItem3;
private System.Windows.Forms.MenuItem menuItem4;
private System.ComponentModel.Container components = null;
public ListBoxContextMenu()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.btnDone = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.btnAdd = new System.Windows.Forms.Button();
this.listBox1 = new System.Windows.Forms.ListBox();
this.contextMenu1 = new System.Windows.Forms.ContextMenu();
this.menuItem1 = new System.Windows.Forms.MenuItem();
this.menuItem2 = new System.Windows.Forms.MenuItem();
this.menuItem3 = new System.Windows.Forms.MenuItem();
this.menuItem4 = new System.Windows.Forms.MenuItem();
this.SuspendLayout();
//
// btnDone
//
this.btnDone.Location = new System.Drawing.Point(208, 16);
this.btnDone.Name = "btnDone";
this.btnDone.TabIndex = 3;
this.btnDone.Text = "Done";
this.btnDone.Click += new System.EventHandler(this.btnDone_Click);
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(16, 88);
this.textBox1.Name = "textBox1";
this.textBox1.TabIndex = 5;
this.textBox1.Text = "";
//
// btnAdd
//
this.btnAdd.Location = new System.Drawing.Point(16, 120);
this.btnAdd.Name = "btnAdd";
this.btnAdd.TabIndex = 6;
this.btnAdd.Text = "Add";
this.btnAdd.Click += new System.EventHandler(this.btnAdd_Click);
//
// listBox1
//
this.listBox1.ContextMenu = this.contextMenu1;
this.listBox1.Location = new System.Drawing.Point(16, 8);
this.listBox1.Name = "listBox1";
this.listBox1.Size = new System.Drawing.Size(120, 69);
this.listBox1.TabIndex = 7;
//
// contextMenu1
//
this.contextMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItem1,
this.menuItem2,
this.menuItem3,
this.menuItem4});
//
// menuItem1
//
this.menuItem1.Index = 0;
this.menuItem1.Text = "Move Down";
this.menuItem1.Click += new System.EventHandler(this.ctxtMenuClick);
//
// menuItem2
//
this.menuItem2.Index = 1;
this.menuItem2.Text = "Move up";
this.menuItem2.Click += new System.EventHandler(this.ctxtMenuClick);
//
// menuItem3
//
this.menuItem3.Index = 2;
this.menuItem3.Text = "Delete";
this.menuItem3.Click += new System.EventHandler(this.ctxtMenuClick);
//
// menuItem4
//
this.menuItem4.Index = 3;
this.menuItem4.Text = "Duplicate";
this.menuItem4.Click += new System.EventHandler(this.ctxtMenuClick);
//
// ListBoxContextMenu
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.listBox1,
this.btnAdd,
this.textBox1,
this.btnDone});
this.Name = "ListBoxContextMenu";
this.ResumeLayout(false);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new ListBoxContextMenu());
}
private void btnDone_Click(object sender, System.EventArgs e)
{
Application.Exit();
}
private void btnAdd_Click(object sender, System.EventArgs e)
{
listBox1.Items.Add(textBox1.Text);
textBox1.Text = "";
}
private void ctxtMenuClick(object sender, System.EventArgs e)
{
if ( listBox1.SelectedIndex != -1 )
{
MenuItem mi = (MenuItem) sender;
MessageBox.Show(mi.Text + " on " + listBox1.SelectedItem,"Context Menu", MessageBoxButtons.OK, MessageBoxIcon.Asterisk);
}else{
MessageBox.Show("Please select an item","Context Menu Tester", MessageBoxButtons.OK, MessageBoxIcon.Asterisk);
}
}
}