Link two DataTable in a DataGrid : DataGrid « ADO.Net « C# / CSharp Tutorial
- C# / CSharp Tutorial
- ADO.Net
- DataGrid
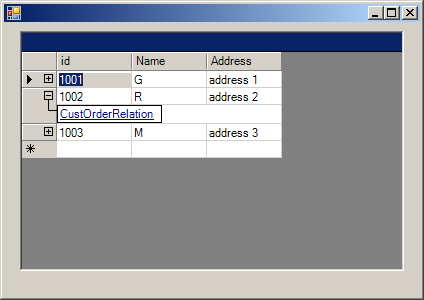
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.Common;
public class DataTableLinkinDataGrid : System.Windows.Forms.Form
{
private System.Windows.Forms.DataGrid dataGrid1;
private System.Data.DataSet dtSet;
public DataTableLinkinDataGrid()
{
this.dataGrid1 = new System.Windows.Forms.DataGrid();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).BeginInit();
this.SuspendLayout();
//
this.dataGrid1.DataMember = "";
this.dataGrid1.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGrid1.Location = new System.Drawing.Point(16, 8);
this.dataGrid1.Size = new System.Drawing.Size(384, 240);
//
// DataTableLinkinDataGrid
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(416, 273);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.dataGrid1});
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).EndInit();
this.ResumeLayout(false);
CreateCustomersTable();
CreateOrdersTable();
BindData();
}
[STAThread]
static void Main()
{
Application.Run(new DataTableLinkinDataGrid());
}
private void CreateCustomersTable()
{
System.Data.DataTable custTable = new DataTable("Customers");
DataColumn dtColumn;
DataRow myDataRow;
dtColumn = new DataColumn();
dtColumn.DataType = System.Type.GetType("System.Int32");
dtColumn.ColumnName = "id";
dtColumn.Caption = "Cust ID";
dtColumn.ReadOnly = true;
dtColumn.Unique = true;
custTable.Columns.Add(dtColumn);
dtColumn = new DataColumn();
dtColumn.DataType = System.Type.GetType("System.String");
dtColumn.ColumnName = "Name";
dtColumn.Caption = "Cust Name";
dtColumn.AutoIncrement = false;
dtColumn.ReadOnly = false;
dtColumn.Unique = false;
custTable.Columns.Add(dtColumn);
dtColumn = new DataColumn();
dtColumn.DataType = System.Type.GetType("System.String");
dtColumn.ColumnName = "Address";
dtColumn.Caption = "Address";
dtColumn.ReadOnly = false;
dtColumn.Unique = false;
custTable.Columns.Add(dtColumn);
DataColumn[] PrimaryKeyColumns = new DataColumn[1];
PrimaryKeyColumns[0] = custTable.Columns["id"];
custTable.PrimaryKey = PrimaryKeyColumns;
dtSet = new DataSet("Customers");
dtSet.Tables.Add(custTable);
myDataRow = custTable.NewRow();
myDataRow["id"] = 1001;
myDataRow["Address"] = "address 1";
myDataRow["Name"] = "G";
custTable.Rows.Add(myDataRow);
myDataRow = custTable.NewRow();
myDataRow["id"] = 1002;
myDataRow["Name"] = "R";
myDataRow["Address"] = "address 2";
custTable.Rows.Add(myDataRow);
myDataRow = custTable.NewRow();
myDataRow["id"] = 1003;
myDataRow["Name"] = "M";
myDataRow["Address"] = "address 3";
custTable.Rows.Add(myDataRow);
}
private void CreateOrdersTable()
{
DataTable ordersTable = new DataTable("Orders");
DataColumn dtColumn;
DataRow dtRow;
dtColumn = new DataColumn();
dtColumn.DataType= System.Type.GetType("System.Int32");
dtColumn.ColumnName = "OrderId";
dtColumn.AutoIncrement = true;
dtColumn.Caption = "Order ID";
dtColumn.ReadOnly = true;
dtColumn.Unique = true;
ordersTable.Columns.Add(dtColumn);
dtColumn = new DataColumn();
dtColumn.DataType= System.Type.GetType("System.String");
dtColumn.ColumnName = "Name";
dtColumn.Caption = "Item Name";
ordersTable.Columns.Add(dtColumn);
// Create CustId column which reprence CustId from
// the custTable
dtColumn = new DataColumn();
dtColumn.DataType= System.Type.GetType("System.Int32");
dtColumn.ColumnName = "CustId";
dtColumn.AutoIncrement = false;
dtColumn.Caption = "CustId";
dtColumn.ReadOnly = false;
dtColumn.Unique = false;
ordersTable.Columns.Add(dtColumn);
dtColumn = new DataColumn();
dtColumn.DataType= System.Type.GetType("System.String");
dtColumn.ColumnName = "Description";
dtColumn.Caption = "Description Name";
ordersTable.Columns.Add(dtColumn);
dtSet.Tables.Add(ordersTable);
dtRow = ordersTable.NewRow();
dtRow["OrderId"] = 0;
dtRow["Name"] = "Book";
dtRow["CustId"] = 1001 ;
dtRow["Description"] = "desc 1" ;
ordersTable.Rows.Add(dtRow);
dtRow = ordersTable.NewRow();
dtRow["OrderId"] = 1;
dtRow["Name"] = "Book";
dtRow["CustId"] = 1001 ;
dtRow["Description"] = "desc 2" ;
ordersTable.Rows.Add(dtRow);
}
private void BindData()
{
DataRelation dtRelation;
DataColumn CustCol = dtSet.Tables["Customers"].Columns["id"];
DataColumn orderCol = dtSet.Tables["Orders"].Columns["CustId"];
dtRelation = new DataRelation("CustOrderRelation", CustCol, orderCol);
dtSet.Tables["Orders"].ParentRelations.Add(dtRelation);
dataGrid1.SetDataBinding(dtSet,"Customers");
}
}