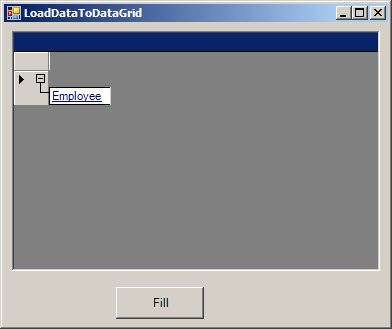
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.SqlClient;
public class LoadDataToDataGrid : System.Windows.Forms.Form
{
private System.Windows.Forms.DataGrid dataGrid1;
private System.Windows.Forms.Button button1;
private System.ComponentModel.Container components = null;
public LoadDataToDataGrid()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.dataGrid1 = new System.Windows.Forms.DataGrid();
this.button1 = new System.Windows.Forms.Button();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).BeginInit();
this.SuspendLayout();
this.dataGrid1.DataMember = "";
this.dataGrid1.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGrid1.Location = new System.Drawing.Point(8, 8);
this.dataGrid1.Name = "dataGrid1";
this.dataGrid1.Size = new System.Drawing.Size(368, 240);
this.dataGrid1.TabIndex = 0;
this.button1.Location = new System.Drawing.Point(112, 264);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(88, 32);
this.button1.TabIndex = 1;
this.button1.Text = "Fill";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// LoadDataToDataGrid
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(384, 302);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.button1,
this.dataGrid1});
this.Name = "LoadDataToDataGrid";
this.Text = "LoadDataToDataGrid";
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).EndInit();
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new LoadDataToDataGrid());
}
private void button1_Click(object sender, System.EventArgs e)
{
string ConnectionString ="server=(local)\\SQLEXPRESS;database=MyDatabase;Integrated Security=SSPI;";
SqlConnection myConnection = new SqlConnection();
myConnection.ConnectionString = ConnectionString;
string sql = "SELECT ID, FirstName FROM Employee";
SqlDataAdapter myAdapter = new SqlDataAdapter(sql, myConnection);
DataSet myDataSet = new DataSet("Employee");
myAdapter.Fill(myDataSet, "Employee");
dataGrid1.DataSource = myDataSet.DefaultViewManager;
}
}