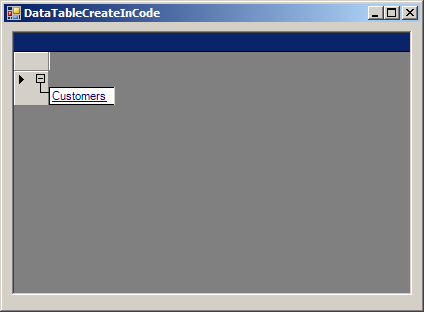
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class DataTableCreateInCode : System.Windows.Forms.Form
{
private System.Windows.Forms.DataGrid dataGrid1;
private System.ComponentModel.Container components = null;
public DataTableCreateInCode()
{
InitializeComponent();
// dcConstructorsTest();
CreateCustTable();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.dataGrid1 = new System.Windows.Forms.DataGrid();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).BeginInit();
this.SuspendLayout();
//
// dataGrid1
//
this.dataGrid1.DataMember = "";
this.dataGrid1.Location = new System.Drawing.Point(8, 8);
this.dataGrid1.Name = "dataGrid1";
this.dataGrid1.Size = new System.Drawing.Size(400, 264);
this.dataGrid1.TabIndex = 0;
//
// DataTableCreateInCode
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(416, 285);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.dataGrid1});
this.Name = "DataTableCreateInCode";
this.Text = "DataTableCreateInCode";
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).EndInit();
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new DataTableCreateInCode());
}
private void dcConstructorsTest()
{
// Create Customers table
DataTable custTable = new DataTable("Customers");
DataSet dtSet = new DataSet();
// Create Price Column
System.Type myDataType;
myDataType = System.Type.GetType("System.Int32");
DataColumn priceCol = new DataColumn("Price", myDataType );
priceCol.Caption = "Price";
custTable.Columns.Add(priceCol);
// Create Quantity Column
DataColumn qtCol = new DataColumn("Quantity");
qtCol.DataType = System.Type.GetType("System.Int32");
qtCol.Caption = "Quantity";
custTable.Columns.Add(qtCol);
// Creating an expression
string strExpr = "Price * Quantity";
// Create Total Column, which is result of Price*Quantity
DataColumn totCol = new DataColumn("Total", myDataType, strExpr, MappingType.Attribute);
totCol.Caption = "Total";
// Add Name column to the table.
custTable.Columns.Add(totCol);
// Add custTable to DataSet
dtSet.Tables.Add(custTable);
// Bind dataset to the data grid
dataGrid1.SetDataBinding(dtSet,"Customers");
}
// Create a DataTable
private void CreateCustTable()
{
// Create a new DataTable
DataTable custTable = new DataTable("Customers");
// Create ID Column
DataColumn IdCol = new DataColumn();
IdCol.ColumnName= "ID";
IdCol.DataType = Type.GetType("System.Int32");
IdCol.ReadOnly = true;
IdCol.AllowDBNull = false;
IdCol.Unique = true;
IdCol.AutoIncrement = true;
IdCol.AutoIncrementSeed = 1;
IdCol.AutoIncrementStep = 1;
custTable.Columns.Add(IdCol);
// Create Name Column
DataColumn nameCol = new DataColumn();
nameCol.ColumnName= "Name";
nameCol.DataType = Type.GetType("System.String");
custTable.Columns.Add(nameCol);
// Create Address Column
DataColumn addCol = new DataColumn();
addCol.ColumnName= "Address";
addCol.DataType = Type.GetType("System.String");
custTable.Columns.Add(addCol);
// Create DOB Column
DataColumn dobCol = new DataColumn();
dobCol.ColumnName= "DOB";
dobCol.DataType = Type.GetType("System.DateTime");
custTable.Columns.Add(dobCol);
// VAR Column
DataColumn fullTimeCol = new DataColumn();
fullTimeCol.ColumnName= "VAR";
fullTimeCol.DataType = Type.GetType("System.Boolean");
custTable.Columns.Add(fullTimeCol);
// Make the ID column the primary key column.
DataColumn[] PrimaryKeyColumns = new DataColumn[1];
PrimaryKeyColumns[0] = custTable.Columns["ID"];
custTable.PrimaryKey = PrimaryKeyColumns;
// Create a dataset
DataSet ds = new DataSet("Customers");
// Add Customers table to the dataset
ds.Tables.Add(custTable);
// Attach the data set to a DataGrid
dataGrid1.DataSource = ds.DefaultViewManager;
}
}