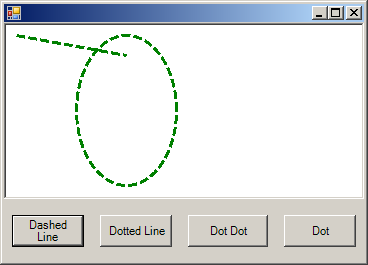
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class PenStyle
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Class Form1
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents PictureBox1 As System.Windows.Forms.PictureBox
Friend WithEvents Button1 As System.Windows.Forms.Button
Friend WithEvents Button2 As System.Windows.Forms.Button
Friend WithEvents Button3 As System.Windows.Forms.Button
Friend WithEvents Button4 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.PictureBox1 = New System.Windows.Forms.PictureBox
Me.Button1 = New System.Windows.Forms.Button
Me.Button2 = New System.Windows.Forms.Button
Me.Button3 = New System.Windows.Forms.Button
Me.Button4 = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'PictureBox1
'
Me.PictureBox1.BackColor = System.Drawing.SystemColors.Window
Me.PictureBox1.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.PictureBox1.Dock = System.Windows.Forms.DockStyle.Top
Me.PictureBox1.Location = New System.Drawing.Point(0, 0)
Me.PictureBox1.Name = "PictureBox1"
Me.PictureBox1.Size = New System.Drawing.Size(360, 176)
Me.PictureBox1.TabIndex = 0
Me.PictureBox1.TabStop = False
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(8, 192)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(72, 32)
Me.Button1.TabIndex = 1
Me.Button1.Text = "Dashed Line"
'
'Button2
'
Me.Button2.Location = New System.Drawing.Point(96, 192)
Me.Button2.Name = "Button2"
Me.Button2.Size = New System.Drawing.Size(72, 32)
Me.Button2.TabIndex = 2
Me.Button2.Text = "Dotted Line"
'
'Button3
'
Me.Button3.Location = New System.Drawing.Point(184, 192)
Me.Button3.Name = "Button3"
Me.Button3.Size = New System.Drawing.Size(80, 32)
Me.Button3.TabIndex = 3
Me.Button3.Text = "Dot Dot"
'
'Button4
'
Me.Button4.Location = New System.Drawing.Point(280, 192)
Me.Button4.Name = "Button4"
Me.Button4.Size = New System.Drawing.Size(72, 32)
Me.Button4.TabIndex = 4
Me.Button4.Text = "Dot"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(360, 238)
Me.Controls.Add(Me.Button4)
Me.Controls.Add(Me.Button3)
Me.Controls.Add(Me.Button2)
Me.Controls.Add(Me.Button1)
Me.Controls.Add(Me.PictureBox1)
Me.ResumeLayout(False)
End Sub
Dim pen1 As System.Drawing.Pen
Dim g As System.Drawing.Graphics
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
PictureBox1.Refresh()
g = PictureBox1.CreateGraphics
pen1 = New System.Drawing.Pen(Color.Green, 3)
pen1.DashStyle = Drawing.Drawing2D.DashStyle.Dash
g.DrawEllipse(pen1, 70, 10, 100, 150)
g.DrawLine(pen1, 10, 10, 120, 30)
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
PictureBox1.Refresh()
g = PictureBox1.CreateGraphics
pen1 = New System.Drawing.Pen(Color.Green, 3)
pen1.DashStyle = Drawing.Drawing2D.DashStyle.DashDot
g.DrawEllipse(pen1, 70, 10, 100, 150)
g.DrawLine(pen1, 10, 10, 120, 30)
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
PictureBox1.Refresh()
g = PictureBox1.CreateGraphics
pen1 = New System.Drawing.Pen(Color.Green, 3)
pen1.DashStyle = Drawing.Drawing2D.DashStyle.DashDotDot
g.DrawEllipse(pen1, 70, 10, 100, 150)
g.DrawLine(pen1, 10, 10, 120, 30)
End Sub
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
PictureBox1.Refresh()
g = PictureBox1.CreateGraphics
pen1 = New System.Drawing.Pen(Color.Green, 3)
pen1.DashStyle = Drawing.Drawing2D.DashStyle.Dot
g.DrawEllipse(pen1, 70, 10, 100, 150)
g.DrawLine(pen1, 10, 10, 120, 30)
End Sub
End Class