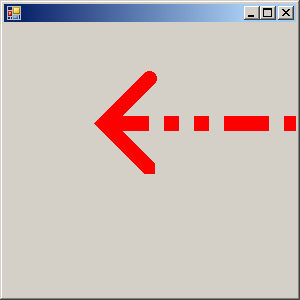
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class SetStrokeCaps
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
public class Form1
Inherits System.Windows.Forms.Form
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
' Create a graphic
Dim g As Graphics = Me.CreateGraphics()
g.Clear(Me.BackColor)
Dim points As Point() = {New Point(-3, -3), New Point(0, 0), New Point(3, -3)}
Dim path As New GraphicsPath
path.AddLines(points)
Dim cap As New CustomLineCap(Nothing, path)
cap.SetStrokeCaps(LineCap.Round, LineCap.Triangle)
Dim redPen As New Pen(Color.Red, 15)
redPen.CustomStartCap = cap
redPen.CustomEndCap = cap
redPen.DashStyle = DashStyle.DashDotDot
g.DrawLine(redPen, New Point(100, 100), New Point(400, 100))
g.Dispose()
End Sub
Public Sub New()
MyBase.New()
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
End Sub
End Class