Sort a List View by Any Column
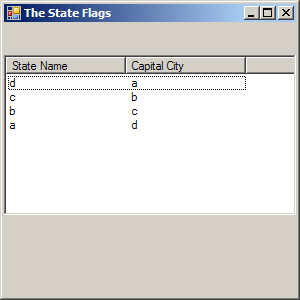
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.ListView listView1;
private System.Windows.Forms.ColumnHeader lvcState;
private System.Windows.Forms.ColumnHeader lvcCapital;
public Form1()
{
InitializeComponent();
listView1.Items.Add (new ListViewItem (new string[]{"a","d"}));
listView1.Items.Add (new ListViewItem (new string[]{"b","c"}));
listView1.Items.Add (new ListViewItem (new string[]{"c","b"}));
listView1.Items.Add (new ListViewItem (new string[]{"d","a"}));
listView1.View = View.Details;
}
private void InitializeComponent()
{
this.listView1 = new System.Windows.Forms.ListView();
this.lvcState = new System.Windows.Forms.ColumnHeader();
this.lvcCapital = new System.Windows.Forms.ColumnHeader();
this.SuspendLayout();
this.listView1.Columns.AddRange(new System.Windows.Forms.ColumnHeader[] {
this.lvcState,
this.lvcCapital});
this.listView1.FullRowSelect = true;
this.listView1.Location = new System.Drawing.Point(0, 32);
this.listView1.MultiSelect = false;
this.listView1.Name = "listView1";
this.listView1.Size = new System.Drawing.Size(292, 160);
this.listView1.Sorting = System.Windows.Forms.SortOrder.Descending;
this.listView1.TabIndex = 3;
this.listView1.ColumnClick += new System.Windows.Forms.ColumnClickEventHandler(this.listView1_OnColumnClick);
this.listView1.SelectedIndexChanged += new System.EventHandler(this.listView1_OnSelectedIndexChanged);
//
// lvcState
//
this.lvcState.Text = "State Name";
this.lvcState.Width = 120;
//
// lvcCapital
//
this.lvcCapital.Text = "Capital City";
this.lvcCapital.Width = 120;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Controls.AddRange(new System.Windows.Forms.Control[] { this.listView1,
});
this.MinimumSize = new System.Drawing.Size(300, 300);
this.Name = "Form1";
this.Text = "The State Flags";
this.ResumeLayout(false);
}
static void Main()
{
Application.Run(new Form1());
}
private void listView1_OnColumnClick(object sender, System.Windows.Forms.ColumnClickEventArgs e)
{
ListViewSorter Sorter = new ListViewSorter();
listView1.ListViewItemSorter = Sorter;
if (!(listView1.ListViewItemSorter is ListViewSorter))
return;
Sorter = (ListViewSorter) listView1.ListViewItemSorter;
if (Sorter.LastSort == e.Column)
{
if (listView1.Sorting == SortOrder.Ascending)
listView1.Sorting = SortOrder.Descending;
else
listView1.Sorting = SortOrder.Ascending;
}
else{
listView1.Sorting = SortOrder.Descending;
}
Sorter.ByColumn = e.Column;
listView1.Sort ();
}
private void listView1_OnSelectedIndexChanged(object sender, System.EventArgs e)
{
if (listView1.SelectedItems.Count == 0)
{
return;
}
string State = listView1.SelectedItems[0].SubItems[0].Text;
string Capital = listView1.SelectedItems[0].SubItems[1].Text;
if (State.Length > 0)
{
Console.WriteLine(State + " " + Capital);
}
}
}
public class ListViewSorter : System.Collections.IComparer
{
public int Compare (object o1, object o2)
{
if (!(o1 is ListViewItem))
return (0);
if (!(o2 is ListViewItem))
return (0);
ListViewItem lvi1 = (ListViewItem) o2;
string str1 = lvi1.SubItems[ByColumn].Text;
ListViewItem lvi2 = (ListViewItem) o1;
string str2 = lvi2.SubItems[ByColumn].Text;
int result;
if (lvi1.ListView.Sorting == SortOrder.Ascending)
result = String.Compare (str1, str2);
else
result = String.Compare (str2, str1);
LastSort = ByColumn;
return (result);
}
public int ByColumn
{
get {return Column;}
set {Column = value;}
}
int Column = 0;
public int LastSort
{
get {return LastColumn;}
set {LastColumn = value;}
}
int LastColumn = 0;
}
Related examples in the same category