Color Yoyo
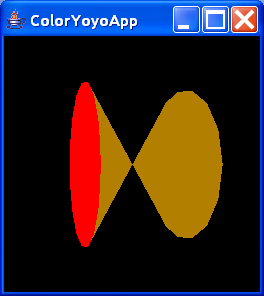
/*
* ColorYoyoApp.java 1.0 98/11/23
*
* Copyright (c) 1998 Sun Microsystems, Inc. All Rights Reserved.
*
* Sun grants you ("Licensee") a non-exclusive, royalty free, license to use,
* modify and redistribute this software in source and binary code form,
* provided that i) this copyright notice and license appear on all copies of
* the software; and ii) Licensee does not utilize the software in a manner
* which is disparaging to Sun.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE POSSIBILITY
* OF SUCH DAMAGES.
*
* This software is not designed or intended for use in on-line control of
* aircraft, air traffic, aircraft navigation or aircraft communications; or in
* the design, construction, operation or maintenance of any nuclear facility.
* Licensee represents and warrants that it will not use or redistribute the
* Software for such purposes.
*/
/*
* Getting Started with the Java 3D API written in Java 3D
*
* This program demonstrates: 1. writing a visual object class In this program,
* Yoyo class defines a visual object by extneding Shape3D. 2. Using
* TriangleFanArray to create surfaces. Four TriangleFan array surfaces are
* contained in an instance of Yoyo. In addition, the color for the Yoyo is set
* in its geometry.
*/
import java.applet.Applet;
import java.awt.BorderLayout;
import java.awt.Frame;
import javax.media.j3d.Alpha;
import javax.media.j3d.BoundingSphere;
import javax.media.j3d.BranchGroup;
import javax.media.j3d.Canvas3D;
import javax.media.j3d.Geometry;
import javax.media.j3d.RotationInterpolator;
import javax.media.j3d.Shape3D;
import javax.media.j3d.Transform3D;
import javax.media.j3d.TransformGroup;
import javax.media.j3d.TriangleFanArray;
import javax.vecmath.Color3f;
import javax.vecmath.Point3d;
import javax.vecmath.Point3f;
import com.sun.j3d.utils.applet.MainFrame;
import com.sun.j3d.utils.universe.SimpleUniverse;
public class ColorYoyoApp extends Applet {
/////////////////////////////////////////////////
//
// create scene graph branch group
//
public class Yoyo extends Shape3D {
////////////////////////////////////////////
//
// create Shape3D with geometry and appearance
// the geometry is created in method yoyoGeometry
// the appearance is created in method yoyoAppearance
//
public Yoyo() {
this.setGeometry(yoyoGeometry());
} // end of Yoyo constructor
////////////////////////////////////////////
//
// create yoyo geometry
// four triangle fans represent the yoyo
// strip indicies_______________
// 0 0N+0 to 1N+0 ( 0 to N )
// 1 1N+1 to 2N+1
// 2 2N+2 to 3N+2
// 3 3N+4 to 4N+3
//
private Geometry yoyoGeometry() {
TriangleFanArray tfa;
int N = 17;
int totalN = 4 * (N + 1);
Point3f coords[] = new Point3f[totalN];
Color3f colors[] = new Color3f[totalN];
Color3f red = new Color3f(1.0f, 0.0f, 0.0f);
Color3f yellow = new Color3f(0.7f, 0.5f, 0.0f);
int stripCounts[] = { N + 1, N + 1, N + 1, N + 1 };
float r = 0.6f;
float w = 0.4f;
int n;
double a;
float x, y;
// set the central points for four triangle fan strips
coords[0 * (N + 1)] = new Point3f(0.0f, 0.0f, w);
coords[1 * (N + 1)] = new Point3f(0.0f, 0.0f, 0.0f);
coords[2 * (N + 1)] = new Point3f(0.0f, 0.0f, 0.0f);
coords[3 * (N + 1)] = new Point3f(0.0f, 0.0f, -w);
colors[0 * (N + 1)] = red;
colors[1 * (N + 1)] = yellow;
colors[2 * (N + 1)] = yellow;
colors[3 * (N + 1)] = red;
for (a = 0, n = 0; n < N; a = 2.0 * Math.PI / (N - 1) * ++n) {
x = (float) (r * Math.cos(a));
y = (float) (r * Math.sin(a));
coords[0 * (N + 1) + n + 1] = new Point3f(x, y, w);
coords[1 * (N + 1) + N - n] = new Point3f(x, y, w);
coords[2 * (N + 1) + n + 1] = new Point3f(x, y, -w);
coords[3 * (N + 1) + N - n] = new Point3f(x, y, -w);
colors[0 * (N + 1) + N - n] = red;
colors[1 * (N + 1) + n + 1] = yellow;
colors[2 * (N + 1) + N - n] = yellow;
colors[3 * (N + 1) + n + 1] = red;
}
tfa = new TriangleFanArray(totalN, TriangleFanArray.COORDINATES
| TriangleFanArray.COLOR_3, stripCounts);
tfa.setCoordinates(0, coords);
tfa.setColors(0, colors);
return tfa;
} // end of method yoyoGeometry in class Yoyo
} // end of class Yoyo
/////////////////////////////////////////////////
//
// create scene graph branch group
//
public BranchGroup createSceneGraph() {
BranchGroup objRoot = new BranchGroup();
// Create the transform group node and initialize it to the
// identity. Enable the TRANSFORM_WRITE capability so that
// our behavior code can modify it at runtime. Add it to the
// root of the subgraph.
TransformGroup objSpin = new TransformGroup();
objSpin.setCapability(TransformGroup.ALLOW_TRANSFORM_WRITE);
objRoot.addChild(objSpin);
objSpin.addChild(new Yoyo());
// Create a new Behavior object that will perform the desired
// operation on the specified transform object and add it into
// the scene graph.
Transform3D yAxis = new Transform3D();
Alpha rotationAlpha = new Alpha(-1, 4000);
RotationInterpolator rotator = new RotationInterpolator(rotationAlpha,
objSpin);
BoundingSphere bounds = new BoundingSphere(new Point3d(0.0, 0.0, 0.0),
100.0);
rotator.setSchedulingBounds(bounds);
objSpin.addChild(rotator);
// Let Java 3D perform optimizations on this scene graph.
objRoot.compile();
return objRoot;
} // end of CreateSceneGraph method of ColorYoyoApp
// Create a simple scene and attach it to the virtual universe
public ColorYoyoApp() {
setLayout(new BorderLayout());
Canvas3D canvas3D = new Canvas3D(null);
add("Center", canvas3D);
BranchGroup scene = createSceneGraph();
// SimpleUniverse is a Convenience Utility class
SimpleUniverse simpleU = new SimpleUniverse(canvas3D);
// This will move the ViewPlatform back a bit so the
// objects in the scene can be viewed.
simpleU.getViewingPlatform().setNominalViewingTransform();
simpleU.addBranchGraph(scene);
} // end of ColorYoyoApp constructor
// The following allows this to be run as an application
// as well as an applet
public static void main(String[] args) {
Frame frame = new MainFrame(new ColorYoyoApp(), 256, 256);
} // end of main method of ColorYoyoApp
} // end of class ColorYoyoApp
Related examples in the same category