Creating Custom Tags
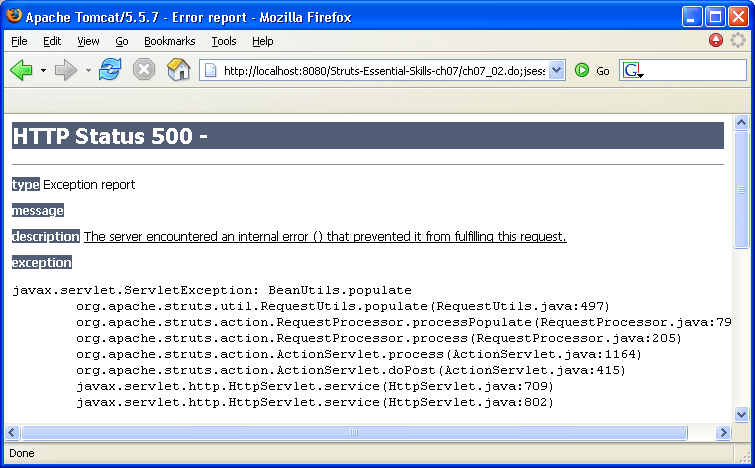
/*
Title: Struts : Essential Skills (Essential Skills)
Authors: Steven Holzner
Publisher: McGraw-Hill Osborne Media
ISBN: 0072256591
*/
//ch07_01.jsp
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<html:html>
<head>
<title>Using <logic> Tags</title>
</head>
<body>
<h1>Using <logic> Tags</h1>
<html:form action="ch07_02.do">
<h2>Enter your data:</h2>
<html:text property="text"/>
<br>
<br>
<html:submit value="Submit"/>
<html:cancel/>
</html:form>
</body>
</html:html>
//ch07_04.jsp
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<HTML>
<HEAD>
<TITLE>Here's Your Data...</TITLE>
</HEAD>
<BODY>
<H1>Here's Your Data...</H1>
<h2>The text field text:</h2>
<bean:write name="ch07_03" property="text"/>
<BR>
<h2>Using <logic:empty name="ch07_03" property="empty"></h2>
<logic:empty name="ch07_03" property="empty">
Results: Empty
</logic:empty>
<BR>
<h2>Using <logic:notEmpty name="ch07_03" property="text"></h2>
<logic:notEmpty name="ch07_03" property="text">
Results: Not empty
</logic:notEmpty>
<BR>
<h2>Using <logic:equal name="ch07_03" property="number" value="6"></h2>
<logic:equal name="ch07_03" property="number" value="6">
Results: Equal
</logic:equal>
<BR>
<h2>Using <logic:notEqual> name="ch07_03" property="number" value="7"</h2>
<logic:notEqual name="ch07_03" property="number" value="7">
Results: Not equal
</logic:notEqual>
<BR>
<h2>Using <logic:greaterEqual name="ch07_03" property="number" value="3"></h2>
<logic:greaterEqual name="ch07_03" property="number" value="3">
Results: Greater than or equal
</logic:greaterEqual>
<BR>
<h2>Using <logic:greaterThan name="ch07_03" property="number" value="4"></h2>
<logic:greaterThan name="ch07_03" property="number" value="4">
Results: Greater than
</logic:greaterThan>
<BR>
<h2>Using <logic:lessEqual name="ch07_03" property="number" value="8"></h2>
<logic:lessEqual name="ch07_03" property="number" value="8">
Results: Less than or equal
</logic:lessEqual>
<BR>
<h2>Using <logic:lessThan name="ch07_03" property="number" value="8"></h2>
<logic:lessThan name="ch07_03" property="number" value="8">
Results: Less than
</logic:lessThan>
<BR>
<h2>Using <logic:match name="ch07_03" property="text" value="6"></h2>
<logic:match name="ch07_03" property="text" value="6">
Results: Matched
</logic:match>
<BR>
<h2>Using <logic:notMatch name="ch07_03" property="number" value="9"></h2>
<logic:notMatch name="ch07_03" property="number" value="9">
Results: No match
</logic:notMatch>
<BR>
<h2>Using <logic:present name="ch07_03" property="number"></h2>
<logic:present name="ch07_03" property="number">
Results: Present
</logic:present>
<BR>
<h2>Using <logic:notPresent name="ch07_03" property="fish"></h2>
<logic:notPresent name="ch07_03" property="fish">
Results: Not present
</logic:notPresent>
<BR>
</BODY>
</HTML>
//ch07_05.jsp
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<html:html>
<head>
<title>Using <bean> Tags</title>
</head>
<body>
<h1>Using <bean> Tags</h1>
<%
Cookie cookie1 = new Cookie("message", "Hello!");
cookie1.setMaxAge(24 * 60 * 60);
response.addCookie(cookie1);
%>
<html:form action="ch07_06.do">
<h2>Enter your data:</h2>
<html:text property="text"/>
<br>
<br>
<html:submit value="Submit"/>
<html:cancel/>
</html:form>
</body>
</html:html>
//ch07_08.jsp
<%@ page import="ch07.ch07_07" %>
<%@ taglib uri="/tags/struts-bean" prefix="bean" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<HTML>
<HEAD>
<TITLE>Here's Your Data...</TITLE>
</HEAD>
<BODY>
<H1>Here's Your Data...</H1>
<h2>The text field text:</h2>
<bean:write name="ch07_07" property="text"/>
<BR>
<h2>The cookie data:</h2>
<bean:cookie id="messageCookie" name="message"/>
<%= messageCookie.getValue() %>
<BR>
<h2>The new variable:</h2>
<bean:define id="variable" name="ch07_07" property="text"/>
<%= variable %>
<BR>
<h2>The user-agent header data:</h2>
<bean:header id="headerObject" name="user-agent"/>
<%= headerObject %>
<BR>
<h2>The parameter data:</h2>
<bean:parameter id="text" name="text"/>
<%= text %>
<BR>
<h2>The mapping data:</h2>
<bean:struts id="mapping" mapping="/ch07_06"/>
<% String[] a = mapping.findForwards();
out.println(a[0]); %>
<BR>
</BODY>
</html>
package ch07;
import org.apache.struts.action.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ch07_03 extends ActionForm
{
private String empty = "";
private String text = "";
private int number;
public String getEmpty()
{
return empty;
}
public void setEmpty(String text)
{
}
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
this.number = Integer.parseInt(text);
}
public int getNumber()
{
return number;
}
public void setNumber(int number)
{
this.number = number;
}
}
package ch07;
import java.io.*;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
public class ch07_06 extends Action
{
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
return mapping.findForward("success");
}
}
package ch07;
import org.apache.struts.action.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ch07_07 extends ActionForm
{
private String text = "";
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
}
}
package ch07;
import java.io.*;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
public class ch07_02 extends Action
{
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
return mapping.findForward("success");
}
}
Struts-Essential-Skills-ch07.zip( 1,456 k)Related examples in the same category