Web Services and the Validator and Tile Packages
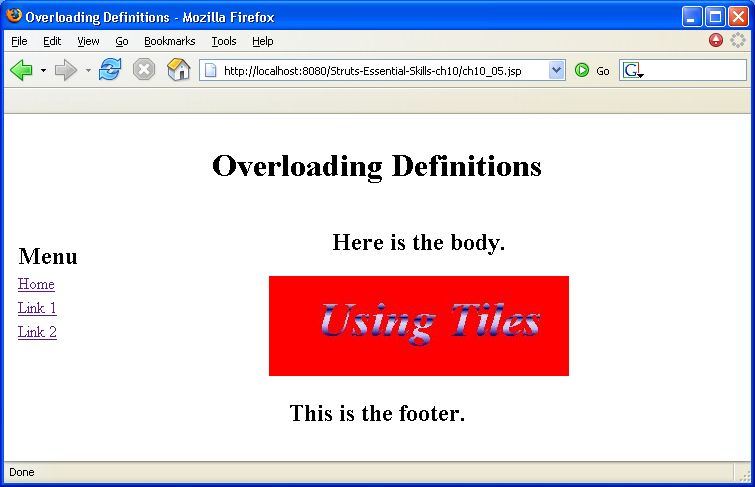
/*
Title: Struts : Essential Skills (Essential Skills)
Authors: Steven Holzner
Publisher: McGraw-Hill Osborne Media
ISBN: 0072256591
*/
//ch10_01.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert page="Layout.jsp" flush="true">
<tiles:put name="title" value="Using Tiles" />
<tiles:put name="header" value="header.jsp" />
<tiles:put name="footer" value="footer.jsp" />
<tiles:put name="menu" value="menu.jsp" />
<tiles:put name="body" value="body.jsp" />
</tiles:insert>
//ch10_02.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert page="Layout.jsp" flush="true">
<tiles:put name="title" value="Using putList" />
<tiles:put name="header" value="header.jsp" />
<tiles:put name="footer" value="footer.jsp" />
<tiles:put name="menu" value="menu.jsp" />
<tiles:put name="body" value="list.jsp" />
</tiles:insert>
//ch10_03.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:definition id="theDefinition" template="/Layout.jsp" >
<tiles:put name="title" value="My first page" />
<tiles:put name="header" value="/header.jsp" />
<tiles:put name="footer" value="/footer.jsp" />
<tiles:put name="menu" value="/menu.jsp" />
<tiles:put name="body" value="/body.jsp" />
</tiles:definition>
<tiles:insert beanName="theDefinition" flush="true" />
//ch10_04.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert definition="tilesDefinition" flush="true" />
//ch10_05.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert definition="tilesDefinition" flush="true" >
<tiles:put name="title" value="Overloading Definitions" />
<tiles:put name="header" value="/overloadedHeader.jsp" />
</tiles:insert>
//ch10_06.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert definition="tilesExtendedDefinition" flush="true" />
//ch10_07.jsp
<%@ taglib uri="/WEB-INF/struts-tiles.tld" prefix="tiles" %>
<tiles:insert definition="attributeDefinition" flush="true" />
//ch10_08.jsp
<%@ taglib uri="/tags/struts-html" prefix="html" %>
<%@ taglib uri="/tags/struts-logic" prefix="logic" %>
<html:html>
<head>
<title>Using <logic> Tags</title>
</head>
<body>
<h1>Using <logic> Tags</h1>
<html:form action="ch10_09.do">
<h2>Enter your data:</h2>
<html:text property="text"/>
<br>
<br>
<html:submit value="Submit"/>
<html:cancel/>
</html:form>
</body>
</html:html>
package ch10;
import java.io.*;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
import org.apache.struts.action.*;
public class ch10_09 extends Action
{
public ActionForward execute(ActionMapping mapping,
ActionForm form,
HttpServletRequest request,
HttpServletResponse response)
throws IOException, ServletException {
return mapping.findForward("success");
}
}
package ch10;
import org.apache.struts.action.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ch10_10 extends ActionForm
{
private String empty = "";
private String text = "";
private int number;
public String getEmpty()
{
return empty;
}
public void setEmpty(String text)
{
}
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
this.number = Integer.parseInt(text);
}
public int getNumber()
{
return number;
}
public void setNumber(int number)
{
this.number = number;
}
}
Struts-Essential-Skills-ch10.zip( 1,451 k)Related examples in the same category