Capture Item Events
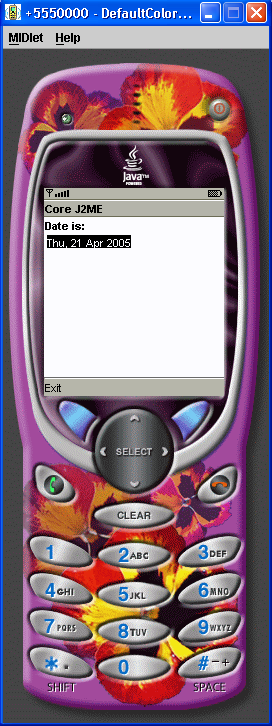
/*--------------------------------------------------
* CaptureItemEvents.java
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class CaptureItemEvents extends MIDlet implements ItemStateListener, CommandListener
{
private Display display; // Reference to Display object for this MIDlet
private Form fmMain; // The main Form
private Command cmExit; // A Command to exit the MIDlet
private DateField dfDate; // Display the date
public CaptureItemEvents()
{
display = Display.getDisplay(this);
// Create the date and populate with current date
dfDate = new DateField("Date is:", DateField.DATE);
dfDate.setDate(new java.util.Date());
cmExit = new Command("Exit", Command.EXIT, 1);
// Create the Form, add Command and DateField
// listen for events from Command and DateField
fmMain = new Form("Core J2ME");
fmMain.addCommand(cmExit);
fmMain.append(dfDate);
fmMain.setCommandListener(this); // Capture Command events (cmExit)
fmMain.setItemStateListener(this); // Capture Item events (dfDate)
}
// Called by application manager to start the MIDlet.
public void startApp()
{
display.setCurrent(fmMain);
}
public void pauseApp()
{ }
public void destroyApp(boolean unconditional)
{ }
public void commandAction(Command c, Displayable s)
{
if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
public void itemStateChanged(Item item)
{
System.out.println("Inside itemStateChanged()");
dfDate.setLabel("New Date: ");
}
}
Related examples in the same category