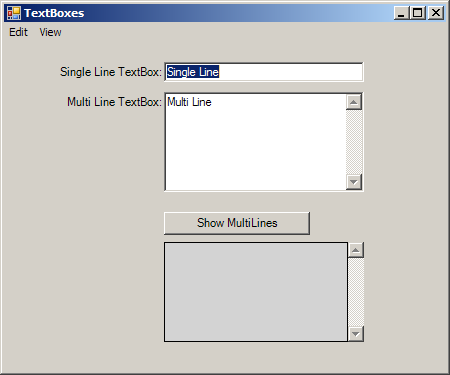
'Programming .Net Windows Applications [ILLUSTRATED] (Paperback)
'by Jesse Liberty (Author), Dan Hurwitz (Author)
'# Publisher: O'Reilly Media, Inc.; 1 edition (October 28, 2003)
'# Language: English
'# ISBN-10: 0596003218
'# ISBN-13: 978-0596003210
imports System
imports System.Drawing
imports System.Windows.Forms
imports System.Text
namespace ProgrammingWinApps
public class TextBoxes : inherits Form
dim yDelta as integer
dim yPos as integer = 20
dim txtSingle as TextBox
dim txtMulti as TextBox
dim txtDisplay as TextBox
dim btn as Button
dim txtBoxes(1) as TextBox
public sub New()
Text = "TextBoxes"
Size = new Size(450,375)
dim lblSingle as new Label()
lblSingle.Parent = me
lblSingle.Text = "Single Line TextBox:"
lblSingle.Location = new Point(10,yPos)
lblSingle.Size = new Size(150,20)
lblSingle.TextAlign = ContentAlignment.MiddleRight
yDelta = lblSingle.Height + 10
txtSingle = new TextBox()
txtSingle.Parent = me
txtSingle.Text = "Single Line"
txtSingle.Size = new Size(200, txtSingle.PreferredHeight)
txtSingle.Location = new Point(lblSingle.Left + _
lblSingle.Size.Width, yPos)
txtSingle.Multiline = false
txtSingle.BorderStyle = BorderStyle.Fixed3D
dim lblMulti as new Label()
lblMulti.Parent = me
lblMulti.Text = "Multi Line TextBox:"
lblMulti.Location = new Point(10, yPos + yDelta)
lblMulti.Size = new Size(150,20)
lblMulti.TextAlign = ContentAlignment.MiddleRight
txtMulti = new TextBox()
txtMulti.Parent = me
txtMulti.Text = "Multi Line"
txtMulti.Size = new Size(200,100)
txtMulti.Location = new Point(lblMulti.Left + _
lblMulti.Size.Width, yPos + yDelta)
txtMulti.AcceptsTab = true
txtMulti.Multiline = true
txtMulti.BorderStyle = BorderStyle.Fixed3D
txtMulti.ScrollBars = ScrollBars.Vertical
btn = new Button()
btn.Parent = me
btn.Text = "Show MultiLines"
btn.Location = new Point(lblMulti.Left + _
lblMulti.Size.Width, yPos + (5 * yDelta))
AddHandler btn.Click, AddressOf btn_Click
dim xSize as integer = CType((Font.Height * .75) * _
btn.Text.Length, integer)
dim ySize as integer = Font.Height + 10
btn.Size = new Size(xSize, ySize)
txtDisplay = new TextBox()
txtDisplay.Parent = me
txtDisplay.Text = ""
txtDisplay.Size = new Size(200,100)
txtDisplay.Location = new Point(lblMulti.Left + _
lblMulti.Size.Width, yPos + (6 * yDelta))
txtDisplay.Multiline = true
txtDisplay.BorderStyle = BorderStyle.FixedSingle
txtDisplay.BackColor = Color.LightGray
txtDisplay.ScrollBars = ScrollBars.Vertical
txtDisplay.ReadOnly = true
' Fill the array of TextBoxes
txtBoxes(0) = txtSingle
txtBoxes(1) = txtMulti
' Menus
' Edit menu items
dim mnuDash1 as new MenuItem("-")
dim mnuDash2 as new MenuItem("-")
dim mnuUndo as new MenuItem("&Undo", _
new EventHandler(AddressOf mnuUndo_Click), _
Shortcut.CtrlZ)
dim mnuCut as new MenuItem("Cu&t", _
new EventHandler(AddressOf mnuCut_Click), _
Shortcut.CtrlX)
dim mnuCopy as new MenuItem("&Copy", _
new EventHandler(AddressOf mnuCopy_Click), _
Shortcut.CtrlC)
dim mnuPaste as new MenuItem("&Paste", _
new EventHandler(AddressOf mnuPaste_Click), _
Shortcut.CtrlV)
dim mnuDelete as new MenuItem("&Delete", _
new EventHandler(AddressOf mnuDelete_Click))
dim mnuSelectAll as new MenuItem("Select &All", _
new EventHandler(AddressOf mnuSelectAll_Click), _
Shortcut.CtrlA)
dim mnuSelect5 as new MenuItem("Select First &5", _
new EventHandler(AddressOf mnuSelect5_Click), _
Shortcut.Ctrl5)
dim mnuClear as new MenuItem("Clea&r", _
new EventHandler(AddressOf mnuClear_Click))
dim mnuEdit as new MenuItem("&Edit", _
new MenuItem() {mnuUndo, mnuDash1, _
mnuCut, mnuCopy, mnuPaste, mnuDelete, mnuDash2, _
mnuSelectAll, mnuSelect5, mnuClear})
' View Menu items
dim mnuScrollToCaret as new MenuItem("&Scroll to Caret", _
new EventHandler(AddressOf mnuScrollToCaret_Click))
dim mnuView as new MenuItem("&View", _
new MenuItem() {mnuScrollToCaret})
' Main menu
Menu = new MainMenu(new MenuItem() {mnuEdit, mnuView})
end sub ' close for constructor
public shared sub Main()
Application.Run(new TextBoxes())
end sub
private sub mnuUndo_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.CanUndo = true then
txt.Undo()
txt.ClearUndo()
end if
end if
next
end sub
private sub mnuCut_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.SelectedText <> "" then
txt.Cut()
end if
end if
next
end sub
private sub mnuCopy_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.SelectionLength > 0 then
txt.Copy()
end if
end if
next
end sub
private sub mnuPaste_Click(ByVal sender As Object, _
ByVal e As EventArgs)
if Clipboard.GetDataObject().GetDataPresent(DataFormats.Text) = true then
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.SelectionLength > 0 then
if MessageBox.Show( _
"Do you want to overwrite the currently selected text?", _
"Cut & Paste", MessageBoxButtons.YesNo) = _
DialogResult.No then
txt.SelectionStart = txt.SelectionStart + _
txt.SelectionLength
end if
end if
txt.Paste()
end if
next
end if
end sub
private sub mnuDelete_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.SelectionLength > 0 then
txt.SelectedText = ""
end if
end if
next
end sub
private sub mnuClear_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
txt.Clear()
end if
next
end sub
private sub mnuSelect5_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
if txt.Text.Length >= 5 then
txt.Select(0,5)
else
txt.Select(0,txt.Text.Length)
end if
end if
next
end sub
private sub mnuSelectAll_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
txt.SelectAll()
end if
next
end sub
private sub mnuScrollToCaret_Click(ByVal sender As Object, _
ByVal e As EventArgs)
dim i as integer
for i = 0 to txtBoxes.Length - 1
if txtBoxes(i).Focused then
dim txt as TextBox = CType(txtBoxes(i), TextBox)
txt.ScrollToCaret()
end if
next
end sub
private sub btn_Click(ByVal sender as object, _
ByVal e as EventArgs)
' Create a string array to hold the Lines property.
dim arLines(txtMulti.Lines.Length - 1) as string
arLines = txtMulti.Lines
' Use stringBuilder for efficiency.
dim str as string = "Line" + vbTab + "String" + vbCrLf
dim sb as new StringBuilder()
sb.Append(str)
' Iterate through the array & display each line.
dim i as integer
for i = 0 to arLines.Length - 1
str = i.ToString() + "." + vbTab + arLines(i) + vbCrLf
sb.Append(str)
next
txtDisplay.Text = sb.ToString()
end sub
end class
end namespace