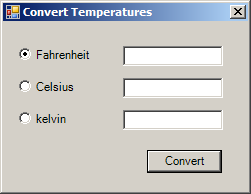
Imports System.Windows.Forms
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class ConvertForm
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.SourceFahrenheit = New System.Windows.Forms.RadioButton
Me.SourceCelsius = New System.Windows.Forms.RadioButton
Me.SourceKelvin = New System.Windows.Forms.RadioButton
Me.ValueFahrenheit = New System.Windows.Forms.TextBox
Me.ValueCelsius = New System.Windows.Forms.TextBox
Me.ValueKelvin = New System.Windows.Forms.TextBox
Me.ConvertTemperature = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'SourceFahrenheit
'
Me.SourceFahrenheit.AutoSize = True
Me.SourceFahrenheit.Checked = True
Me.SourceFahrenheit.Location = New System.Drawing.Point(16, 24)
Me.SourceFahrenheit.Name = "SourceFahrenheit"
Me.SourceFahrenheit.Size = New System.Drawing.Size(75, 17)
Me.SourceFahrenheit.TabIndex = 0
Me.SourceFahrenheit.TabStop = True
Me.SourceFahrenheit.Text = "&Fahrenheit"
Me.SourceFahrenheit.UseVisualStyleBackColor = True
'
'SourceCelsius
'
Me.SourceCelsius.AutoSize = True
Me.SourceCelsius.Location = New System.Drawing.Point(16, 56)
Me.SourceCelsius.Name = "SourceCelsius"
Me.SourceCelsius.Size = New System.Drawing.Size(58, 17)
Me.SourceCelsius.TabIndex = 2
Me.SourceCelsius.Text = "&Celsius"
Me.SourceCelsius.UseVisualStyleBackColor = True
'
'SourceKelvin
'
Me.SourceKelvin.AutoSize = True
Me.SourceKelvin.Location = New System.Drawing.Point(16, 88)
Me.SourceKelvin.Name = "SourceKelvin"
Me.SourceKelvin.Size = New System.Drawing.Size(53, 17)
Me.SourceKelvin.TabIndex = 4
Me.SourceKelvin.Text = "&kelvin"
Me.SourceKelvin.UseVisualStyleBackColor = True
'
'ValueFahrenheit
'
Me.ValueFahrenheit.Location = New System.Drawing.Point(120, 24)
Me.ValueFahrenheit.Name = "ValueFahrenheit"
Me.ValueFahrenheit.Size = New System.Drawing.Size(100, 20)
Me.ValueFahrenheit.TabIndex = 1
'
'ValueCelsius
'
Me.ValueCelsius.Location = New System.Drawing.Point(120, 56)
Me.ValueCelsius.Name = "ValueCelsius"
Me.ValueCelsius.Size = New System.Drawing.Size(100, 20)
Me.ValueCelsius.TabIndex = 3
'
'ValueKelvin
'
Me.ValueKelvin.Location = New System.Drawing.Point(120, 88)
Me.ValueKelvin.Name = "ValueKelvin"
Me.ValueKelvin.Size = New System.Drawing.Size(100, 20)
Me.ValueKelvin.TabIndex = 5
'
'ConvertTemperature
'
Me.ConvertTemperature.Location = New System.Drawing.Point(144, 128)
Me.ConvertTemperature.Name = "ConvertTemperature"
Me.ConvertTemperature.Size = New System.Drawing.Size(75, 23)
Me.ConvertTemperature.TabIndex = 6
Me.ConvertTemperature.Text = "Convert"
Me.ConvertTemperature.UseVisualStyleBackColor = True
'
'ConvertForm
'
Me.AcceptButton = Me.ConvertTemperature
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(245, 169)
Me.Controls.Add(Me.ConvertTemperature)
Me.Controls.Add(Me.ValueKelvin)
Me.Controls.Add(Me.ValueCelsius)
Me.Controls.Add(Me.ValueFahrenheit)
Me.Controls.Add(Me.SourceKelvin)
Me.Controls.Add(Me.SourceCelsius)
Me.Controls.Add(Me.SourceFahrenheit)
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedSingle
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.Name = "ConvertForm"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
Me.Text = "Convert Temperatures"
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents SourceFahrenheit As System.Windows.Forms.RadioButton
Friend WithEvents SourceCelsius As System.Windows.Forms.RadioButton
Friend WithEvents SourceKelvin As System.Windows.Forms.RadioButton
Friend WithEvents ValueFahrenheit As System.Windows.Forms.TextBox
Friend WithEvents ValueCelsius As System.Windows.Forms.TextBox
Friend WithEvents ValueKelvin As System.Windows.Forms.TextBox
Friend WithEvents ConvertTemperature As System.Windows.Forms.Button
End Class
Public Class ConvertForm
Private Sub ConvertTemperature_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ConvertTemperature.Click
On Error Resume Next
If (SourceFahrenheit.Checked = True) Then
If (IsNumeric(ValueFahrenheit.Text) = True) Then
ValueCelsius.Text = _
(Val(ValueFahrenheit.Text) - 32) / 1.8
ValueKelvin.Text = _
((Val(ValueFahrenheit.Text) - 32) / 1.8) + 273.15
Else
ValueCelsius.Text = "Error"
ValueKelvin.Text = "Error"
End If
ElseIf (SourceCelsius.Checked = True) Then
If (IsNumeric(ValueCelsius.Text) = True) Then
ValueFahrenheit.Text = _
(Val(ValueCelsius.Text) * 1.8) + 32
ValueKelvin.Text = Val(ValueCelsius.Text) + 273.15
Else
ValueFahrenheit.Text = "Error"
ValueKelvin.Text = "Error"
End If
Else
If (IsNumeric(ValueKelvin.Text) = True) Then
ValueFahrenheit.Text = _
((Val(ValueKelvin.Text) - 273.15) * 1.8) + 32
ValueCelsius.Text = Val(ValueKelvin.Text) - 273.15
Else
ValueFahrenheit.Text = "Error"
ValueCelsius.Text = "Error"
End If
End If
End Sub
End Class
public class FahrenheitCelsiusConverter
Public Shared Sub Main()
Application.Run(New ConvertForm)
End Sub
End Class