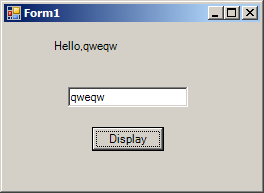
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class GetTextInTextBoxAndSetToLabel
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
Friend WithEvents Button1 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Label1 = New System.Windows.Forms.Label
Me.TextBox1 = New System.Windows.Forms.TextBox
Me.Button1 = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'Label1
'
Me.Label1.Location = New System.Drawing.Point(48, 16)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(160, 32)
Me.Label1.TabIndex = 0
'
'TextBox1
'
Me.TextBox1.Location = New System.Drawing.Point(64, 64)
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(120, 20)
Me.TextBox1.TabIndex = 1
Me.TextBox1.Text = ""
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(88, 104)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(72, 24)
Me.Button1.TabIndex = 2
Me.Button1.Text = "Display"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(256, 166)
Me.Controls.Add(Me.Button1)
Me.Controls.Add(Me.TextBox1)
Me.Controls.Add(Me.Label1)
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Label1.Text = "Hello," + TextBox1.Text + ""
End Sub
End Class