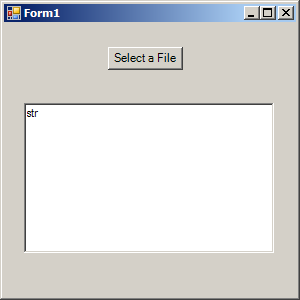
Imports System.IO
Imports System.Windows.Forms
public class TextFileDisplayTextBox
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents Button1 As System.Windows.Forms.Button
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Button1 = New System.Windows.Forms.Button()
Me.TextBox1 = New System.Windows.Forms.TextBox()
Me.SuspendLayout()
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(104, 24)
Me.Button1.Name = "Button1"
Me.Button1.TabIndex = 0
Me.Button1.Text = "Select a File"
'
'TextBox1
'
Me.TextBox1.Location = New System.Drawing.Point(20, 80)
Me.TextBox1.Multiline = True
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(250, 150)
Me.TextBox1.TabIndex = 1
Me.TextBox1.Text = ""
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.Button1, Me.TextBox1})
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub Button1_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim FileDB As New OpenFileDialog()
FileDB.Filter = "All files | *.* | Word files | *.doc | Text files | *.txt"
FileDB.FilterIndex = 3
FileDB.InitialDirectory = "C:\Temp"
If (FileDB.ShowDialog() = DialogResult.OK) Then
Dim FS As FileStream
Try
FS = FileDB.OpenFile()
Catch
MsgBox("Error opening " & FileDB.FileName)
End Try
Dim TextData(1025) As Byte
Dim BytesRead As Integer
Dim I As Integer
Dim NewText As String
Do
Try
BytesRead = FS.Read(TextData, 1, 1024)
NewText = ""
For I = 1 To BytesRead
NewText = NewText & Chr(TextData(I))
Next
TextBox1.AppendText(NewText)
Catch
MsgBox("Error reading file")
End Try
Loop While (BytesRead <> 0)
FS.Close()
Else
MsgBox("User selected Cancel")
End If
End Sub
End Class