Draw rectangle 2
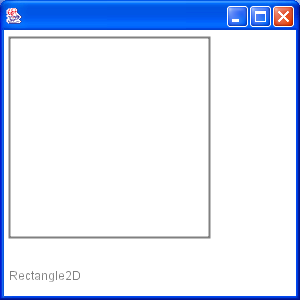
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.GradientPaint;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.geom.Arc2D;
import java.awt.geom.Ellipse2D;
import java.awt.geom.GeneralPath;
import java.awt.geom.Line2D;
import java.awt.geom.Rectangle2D;
import java.awt.geom.RoundRectangle2D;
import javax.swing.JApplet;
import javax.swing.JFrame;
public class RectangleDemo2D extends JApplet {
final static BasicStroke stroke = new BasicStroke(2.0f);
public void init() {
setBackground(Color.white);
setForeground(Color.white);
}
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2.setPaint(Color.gray);
int x = 5;
int y = 7;
g2.setStroke(stroke);
g2.draw(new Rectangle2D.Double(x, y, 200, 200));
g2.drawString("Rectangle2D", x, 250);
}
public static void main(String s[]) {
JFrame f = new JFrame("");
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
JApplet applet = new RectangleDemo2D();
f.getContentPane().add("Center", applet);
applet.init();
f.pack();
f.setSize(new Dimension(300, 300));
f.show();
}
}
Related examples in the same category
1. | Creating Basic Shapes | | |
2. | fillRect (int, int, int, int) method draws a solid rectangle | | |
3. | Creating a Shape Using Lines and Curves | | |
4. | Combining Shapes | | |
5. | Draw rectangles, use the drawRect() method. To fill rectangles, use the fillRect() method | | |
6. | Draw line | | 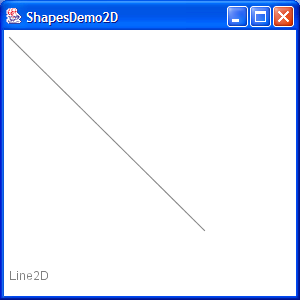 |
7. | Draw a Polygon | | 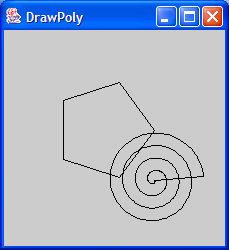 |
8. | Draw an oval outline | | |
9. | Draw a (Round)rectangle | | 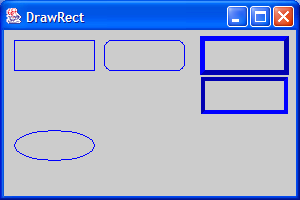 |
10. | Fill a polygon | |  |
11. | Fill a solid oval | | |
12. | Fill a (Round)rectangle | | 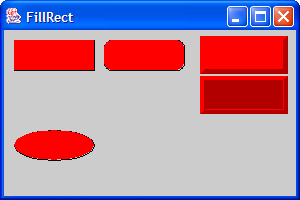 |
13. | Change font | | 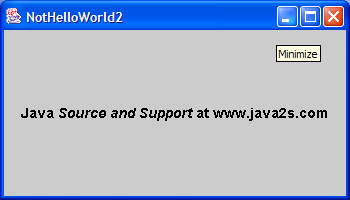 |
14. | Draw Arc | | 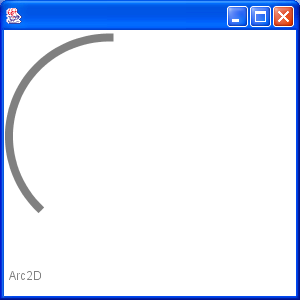 |
15. | Draw Ellipse | | 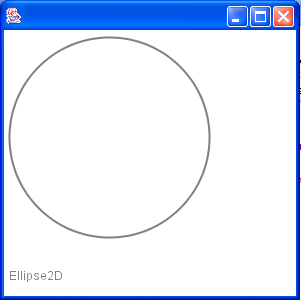 |
16. | Fill a Rectangle 2 | | 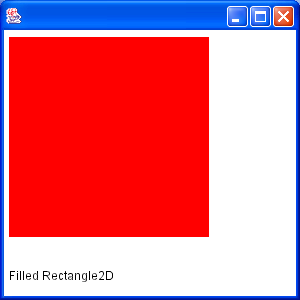 |
17. | Fill Arc 2 | | 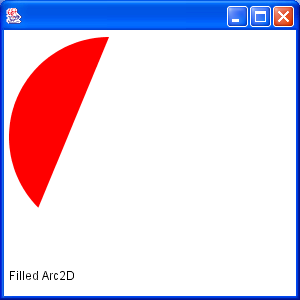 |
18. | Draw text | |  |
19. | Draw unicode string | | 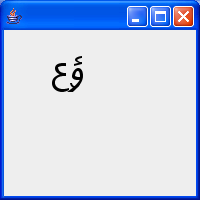 |
20. | Shape combine | | 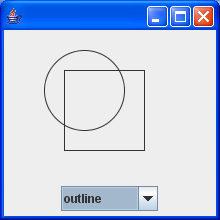 |
21. | Effects | | 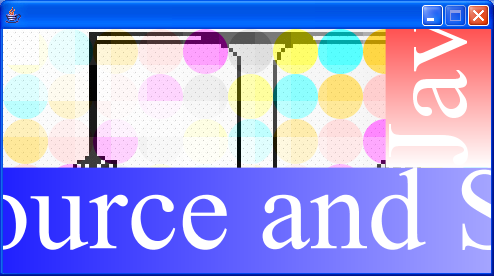 |
22. | Mouse drag and drop to draw | | 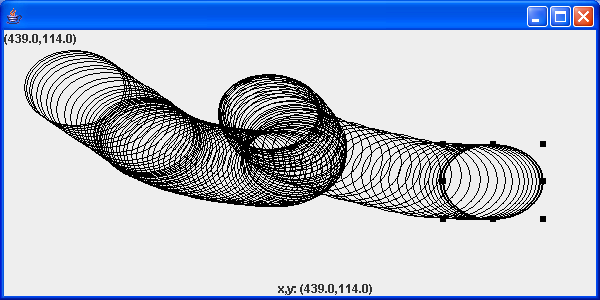 |
23. | Arc demonstration: scale, move, rotate, sheer | | 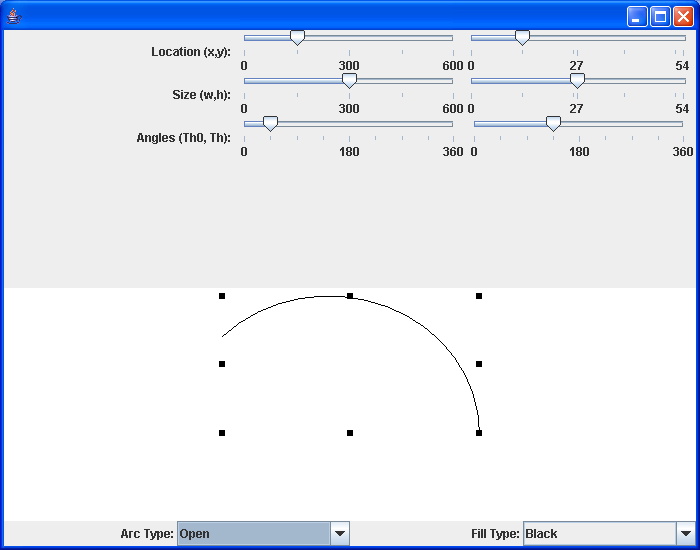 |
24. | Hypnosis Spiral | | 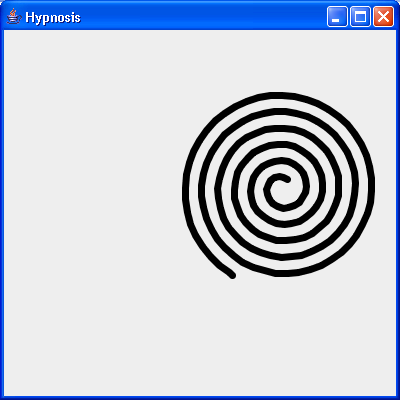 |
25. | GlyphVector.getNumGlyphs() | | |
26. | Resize a shape | | |
27. | Rectangle with rounded corners drawn using Java 2D Graphics API | | |
28. | Compares two ellipses and returns true if they are equal or both null. | | |
29. | Compares two lines are returns true if they are equal or both null. | | |
30. | Creates a diagonal cross shape. | | |
31. | Creates a diamond shape. | | |
32. | Creates a new Stroke-Object for the given type and with. | | |
33. | Creates a region surrounding a line segment by 'widening' the line segment. | | |
34. | Creates a triangle shape that points downwards. | | |
35. | Creates a triangle shape that points upwards. | | |
36. | Generate Polygon | | |
37. | Polygon with float coordinates. | | |
38. | Polyline 2D | | |
39. | Serialises a Shape object. | | |
40. | Tests two polygons for equality. If both are null this method returns true. | | |
41. | Union two rectangles | | |
42. | Calculate Intersection Clip | | |
43. | Draws a shape with the specified rotation about (x, y). | | |
44. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). | | |
45. | Reads a Point2D object that has been serialised by the writePoint2D(Point2D, ObjectOutputStream)} method. | | |
46. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. | | |
47. | RectListManager is a class to manage a list of rectangular regions. | | |
48. | Fill Rectangle2D.Double and Ellipse2D.Double | | 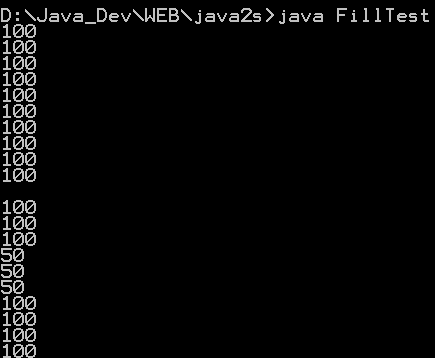 |
49. | This program demonstrates the various 2D shapes | | 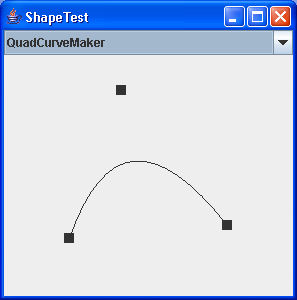 |