Reads a Point2D object that has been serialised by the writePoint2D(Point2D, ObjectOutputStream)} method.
import java.awt.geom.Point2D;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
/*
* JCommon : a free general purpose class library for the Java(tm) platform
*
*
* (C) Copyright 2000-2005, by Object Refinery Limited and Contributors.
*
* Project Info: http://www.jfree.org/jcommon/index.html
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or
* (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301,
* USA.
*
* [Java is a trademark or registered trademark of Sun Microsystems, Inc.
* in the United States and other countries.]
*
* ------------
* IOUtils.java
* ------------
* (C)opyright 2002-2004, by Thomas Morgner and Contributors.
*
* Original Author: Thomas Morgner;
* Contributor(s): David Gilbert (for Object Refinery Limited);
*
* $Id: IOUtils.java,v 1.8 2009/01/22 08:34:58 taqua Exp $
*
* Changes
* -------
* 26-Jan-2003 : Initial version
* 23-Feb-2003 : Documentation
* 25-Feb-2003 : Fixed Checkstyle issues (DG);
* 29-Apr-2003 : Moved to jcommon
* 04-Jan-2004 : Fixed JDK 1.2.2 issues with createRelativeURL;
* added support for query strings within these urls (TM);
*/
public class Main {
/**
* Returns <code>true</code> if a class implements <code>Serializable</code>
* and <code>false</code> otherwise.
*
* @param c the class.
*
* @return A boolean.
*/
public static boolean isSerializable(final Class c) {
/**
final Class[] interfaces = c.getInterfaces();
for (int i = 0; i < interfaces.length; i++) {
if (interfaces[i].equals(Serializable.class)) {
return true;
}
}
Class cc = c.getSuperclass();
if (cc != null) {
return isSerializable(cc);
}
*/
return (Serializable.class.isAssignableFrom(c));
}
/**
* Reads a <code>Point2D</code> object that has been serialised by the
* {@link #writePoint2D(Point2D, ObjectOutputStream)} method.
*
* @param stream the input stream (<code>null</code> not permitted).
*
* @return The point object (possibly <code>null</code>).
*
* @throws IOException if there is an I/O problem.
*/
public static Point2D readPoint2D(final ObjectInputStream stream)
throws IOException {
if (stream == null) {
throw new IllegalArgumentException("Null 'stream' argument.");
}
Point2D result = null;
final boolean isNull = stream.readBoolean();
if (!isNull) {
final double x = stream.readDouble();
final double y = stream.readDouble();
result = new Point2D.Double(x, y);
}
return result;
}
/**
* Serialises a <code>Point2D</code> object.
*
* @param p the point object (<code>null</code> permitted).
* @param stream the output stream (<code>null</code> not permitted).
*
* @throws IOException if there is an I/O error.
*/
public static void writePoint2D(final Point2D p,
final ObjectOutputStream stream)
throws IOException {
if (stream == null) {
throw new IllegalArgumentException("Null 'stream' argument.");
}
if (p != null) {
stream.writeBoolean(false);
stream.writeDouble(p.getX());
stream.writeDouble(p.getY());
}
else {
stream.writeBoolean(true);
}
}
}
Related examples in the same category
1. | Creating Basic Shapes | | |
2. | fillRect (int, int, int, int) method draws a solid rectangle | | |
3. | Creating a Shape Using Lines and Curves | | |
4. | Combining Shapes | | |
5. | Draw rectangles, use the drawRect() method. To fill rectangles, use the fillRect() method | | |
6. | Draw line | | 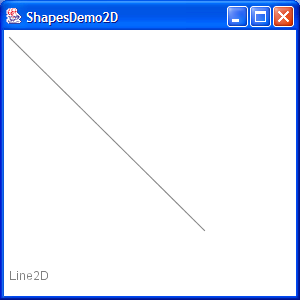 |
7. | Draw a Polygon | | 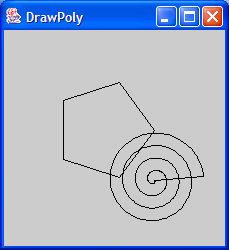 |
8. | Draw an oval outline | | |
9. | Draw a (Round)rectangle | | 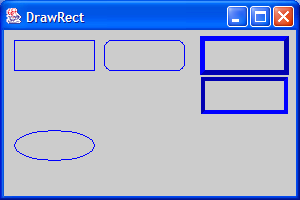 |
10. | Fill a polygon | |  |
11. | Fill a solid oval | | |
12. | Fill a (Round)rectangle | | 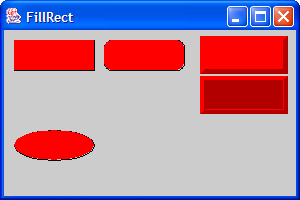 |
13. | Change font | | 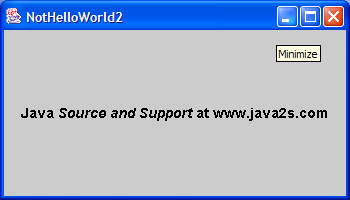 |
14. | Draw rectangle 2 | | 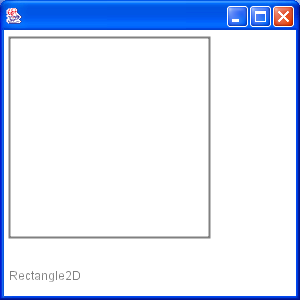 |
15. | Draw Arc | | 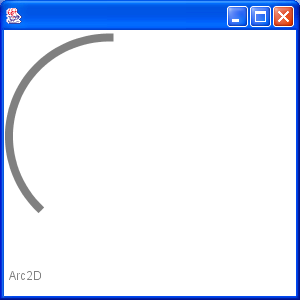 |
16. | Draw Ellipse | | 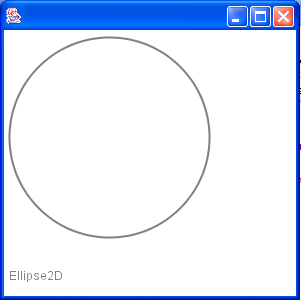 |
17. | Fill a Rectangle 2 | | 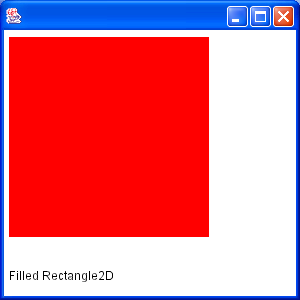 |
18. | Fill Arc 2 | | 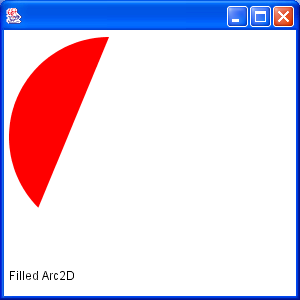 |
19. | Draw text | |  |
20. | Draw unicode string | | 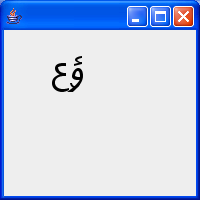 |
21. | Shape combine | | 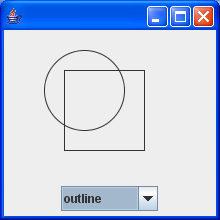 |
22. | Effects | | 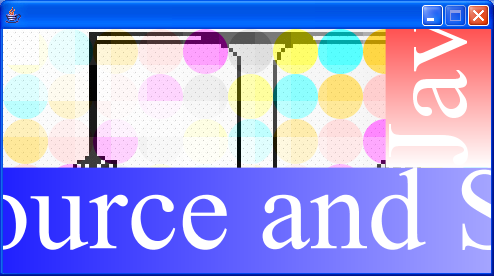 |
23. | Mouse drag and drop to draw | | 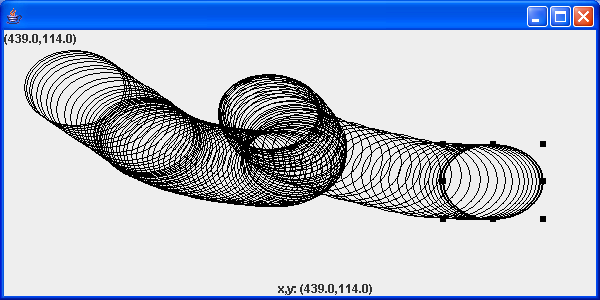 |
24. | Arc demonstration: scale, move, rotate, sheer | | 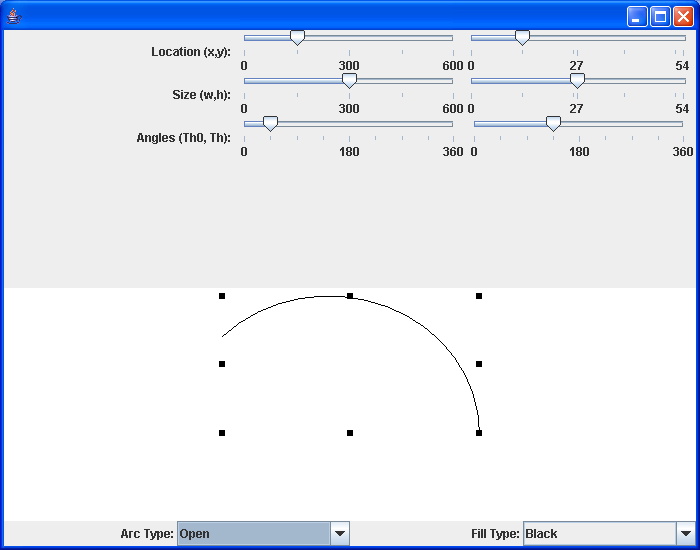 |
25. | Hypnosis Spiral | | 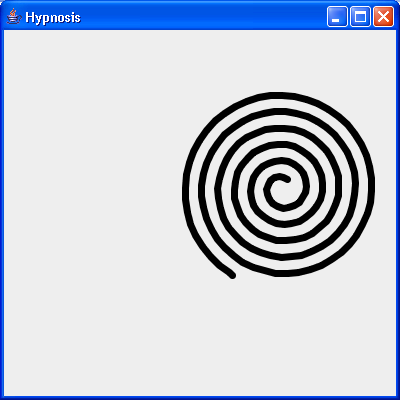 |
26. | GlyphVector.getNumGlyphs() | | |
27. | Resize a shape | | |
28. | Rectangle with rounded corners drawn using Java 2D Graphics API | | |
29. | Compares two ellipses and returns true if they are equal or both null. | | |
30. | Compares two lines are returns true if they are equal or both null. | | |
31. | Creates a diagonal cross shape. | | |
32. | Creates a diamond shape. | | |
33. | Creates a new Stroke-Object for the given type and with. | | |
34. | Creates a region surrounding a line segment by 'widening' the line segment. | | |
35. | Creates a triangle shape that points downwards. | | |
36. | Creates a triangle shape that points upwards. | | |
37. | Generate Polygon | | |
38. | Polygon with float coordinates. | | |
39. | Polyline 2D | | |
40. | Serialises a Shape object. | | |
41. | Tests two polygons for equality. If both are null this method returns true. | | |
42. | Union two rectangles | | |
43. | Calculate Intersection Clip | | |
44. | Draws a shape with the specified rotation about (x, y). | | |
45. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). | | |
46. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. | | |
47. | RectListManager is a class to manage a list of rectangular regions. | | |
48. | Fill Rectangle2D.Double and Ellipse2D.Double | | 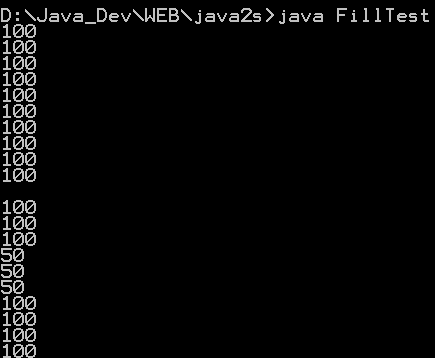 |
49. | This program demonstrates the various 2D shapes | | 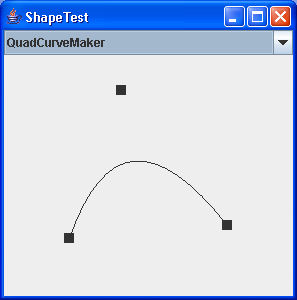 |