Rectangle with rounded corners drawn using Java 2D Graphics API
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.RoundRectangle2D;
import javax.swing.JFrame;
public class Main extends JFrame {
public Main() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 600);
}
public void paint(Graphics g) {
Graphics2D graphics2 = (Graphics2D) g;
RoundRectangle2D roundedRectangle = new RoundRectangle2D.Float(10, 10, 240, 160, 10, 10);
graphics2.draw(roundedRectangle);
}
public static void main(String args[]) {
new Main().setVisible(true);
}
}
Related examples in the same category
1. | Creating Basic Shapes | | |
2. | fillRect (int, int, int, int) method draws a solid rectangle | | |
3. | Creating a Shape Using Lines and Curves | | |
4. | Combining Shapes | | |
5. | Draw rectangles, use the drawRect() method. To fill rectangles, use the fillRect() method | | |
6. | Draw line | | 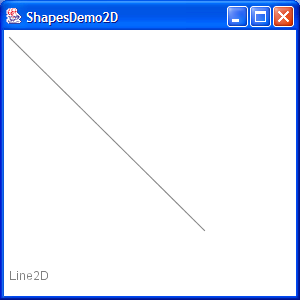 |
7. | Draw a Polygon | | 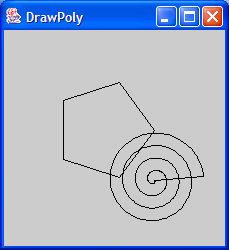 |
8. | Draw an oval outline | | |
9. | Draw a (Round)rectangle | | 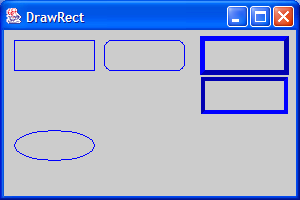 |
10. | Fill a polygon | |  |
11. | Fill a solid oval | | |
12. | Fill a (Round)rectangle | | 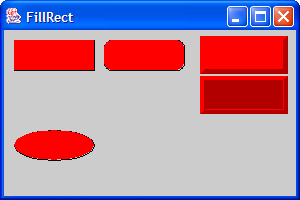 |
13. | Change font | | 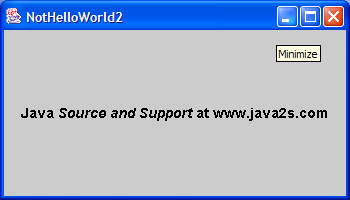 |
14. | Draw rectangle 2 | | 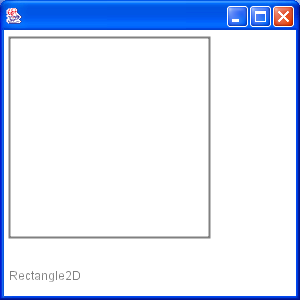 |
15. | Draw Arc | | 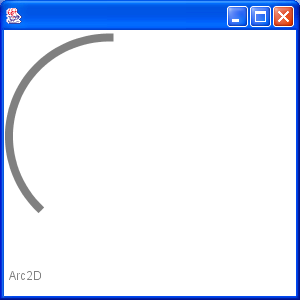 |
16. | Draw Ellipse | | 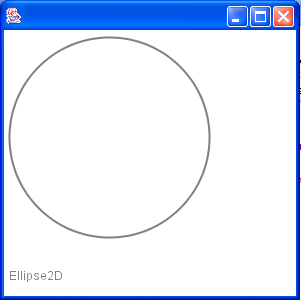 |
17. | Fill a Rectangle 2 | | 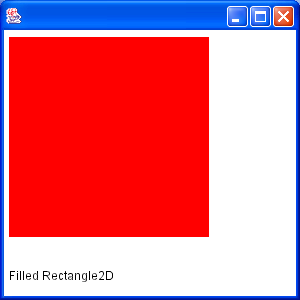 |
18. | Fill Arc 2 | | 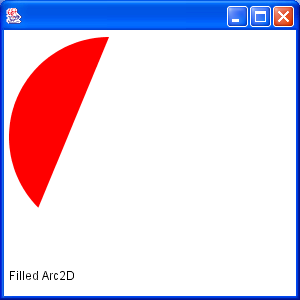 |
19. | Draw text | |  |
20. | Draw unicode string | | 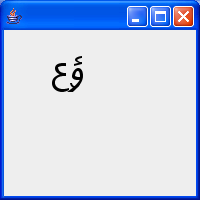 |
21. | Shape combine | | 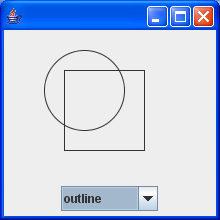 |
22. | Effects | | 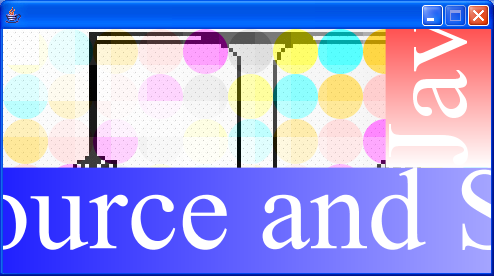 |
23. | Mouse drag and drop to draw | | 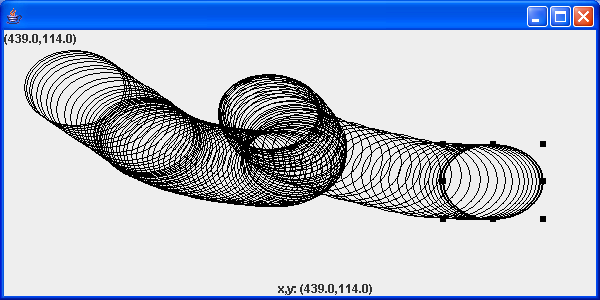 |
24. | Arc demonstration: scale, move, rotate, sheer | | 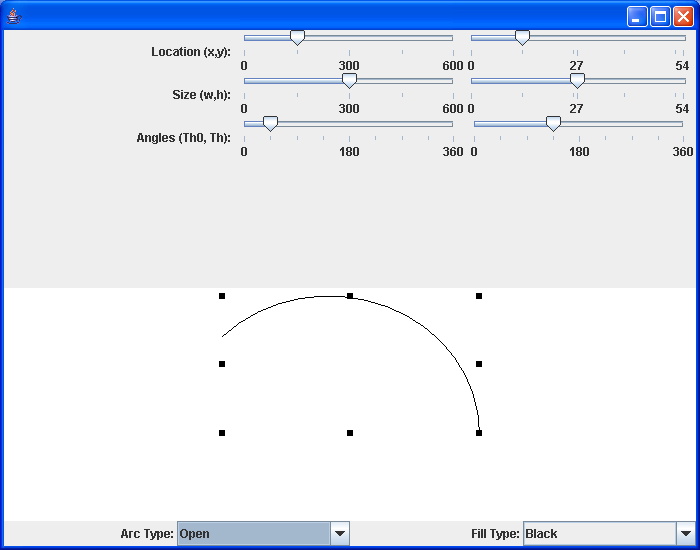 |
25. | Hypnosis Spiral | | 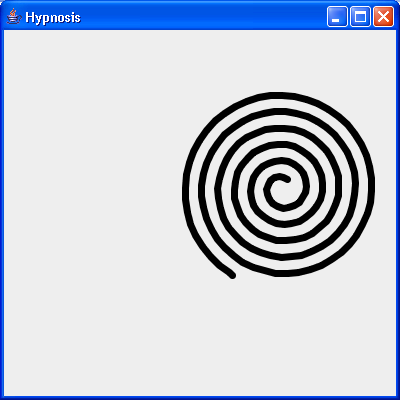 |
26. | GlyphVector.getNumGlyphs() | | |
27. | Resize a shape | | |
28. | Compares two ellipses and returns true if they are equal or both null. | | |
29. | Compares two lines are returns true if they are equal or both null. | | |
30. | Creates a diagonal cross shape. | | |
31. | Creates a diamond shape. | | |
32. | Creates a new Stroke-Object for the given type and with. | | |
33. | Creates a region surrounding a line segment by 'widening' the line segment. | | |
34. | Creates a triangle shape that points downwards. | | |
35. | Creates a triangle shape that points upwards. | | |
36. | Generate Polygon | | |
37. | Polygon with float coordinates. | | |
38. | Polyline 2D | | |
39. | Serialises a Shape object. | | |
40. | Tests two polygons for equality. If both are null this method returns true. | | |
41. | Union two rectangles | | |
42. | Calculate Intersection Clip | | |
43. | Draws a shape with the specified rotation about (x, y). | | |
44. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). | | |
45. | Reads a Point2D object that has been serialised by the writePoint2D(Point2D, ObjectOutputStream)} method. | | |
46. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. | | |
47. | RectListManager is a class to manage a list of rectangular regions. | | |
48. | Fill Rectangle2D.Double and Ellipse2D.Double | | 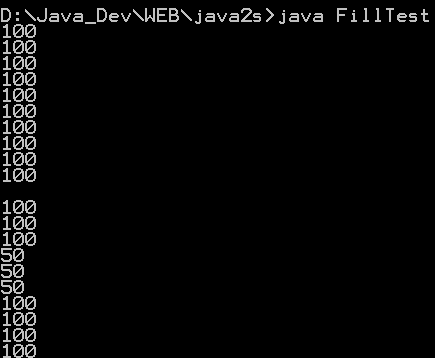 |
49. | This program demonstrates the various 2D shapes | | 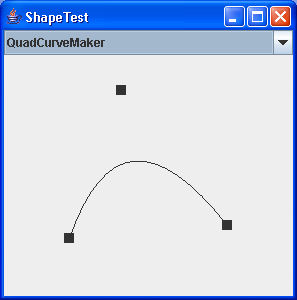 |