Fill Rectangle2D.Double and Ellipse2D.Double
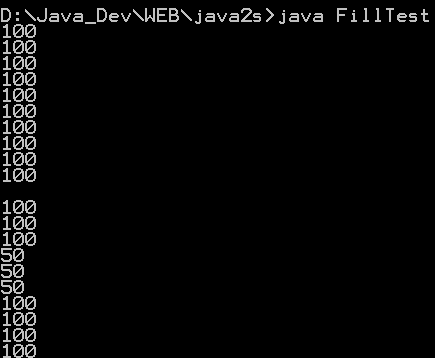
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Rectangle2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
/**
* @version 1.33 2007-04-14
* @author Cay Horstmann
*/
public class FillTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
FillFrame frame = new FillFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
/**
* A frame that contains a component with drawings
*/
class FillFrame extends JFrame
{
public FillFrame()
{
setTitle("FillTest");
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// add component to frame
FillComponent component = new FillComponent();
add(component);
}
public static final int DEFAULT_WIDTH = 400;
public static final int DEFAULT_HEIGHT = 400;
}
/**
* A component that displays filled rectangles and ellipses
*/
class FillComponent extends JComponent
{
public void paintComponent(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
// draw a rectangle
double leftX = 100;
double topY = 100;
double width = 200;
double height = 150;
Rectangle2D rect = new Rectangle2D.Double(leftX, topY, width, height);
g2.setPaint(Color.RED);
g2.fill(rect);
// draw the enclosed ellipse
Ellipse2D ellipse = new Ellipse2D.Double();
ellipse.setFrame(rect);
g2.setPaint(new Color(0, 128, 128)); // a dull blue-green
g2.fill(ellipse);
}
}
Related examples in the same category
1. | Creating Basic Shapes | | |
2. | fillRect (int, int, int, int) method draws a solid rectangle | | |
3. | Creating a Shape Using Lines and Curves | | |
4. | Combining Shapes | | |
5. | Draw rectangles, use the drawRect() method. To fill rectangles, use the fillRect() method | | |
6. | Draw line | | 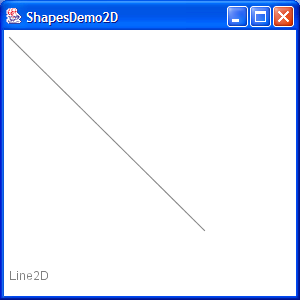 |
7. | Draw a Polygon | | 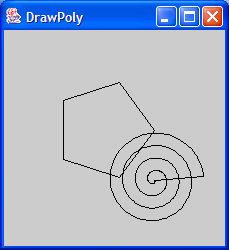 |
8. | Draw an oval outline | | |
9. | Draw a (Round)rectangle | | 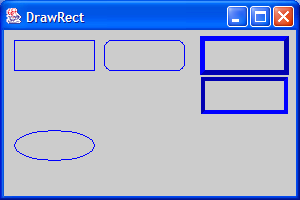 |
10. | Fill a polygon | |  |
11. | Fill a solid oval | | |
12. | Fill a (Round)rectangle | | 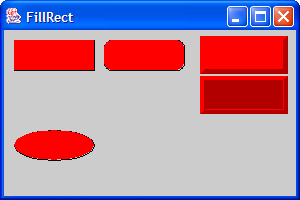 |
13. | Change font | | 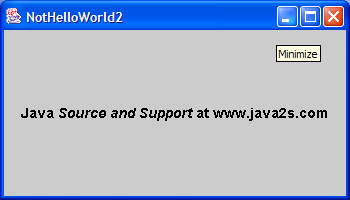 |
14. | Draw rectangle 2 | | 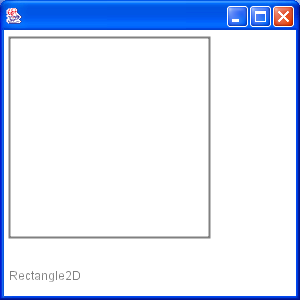 |
15. | Draw Arc | | 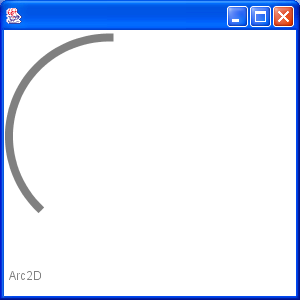 |
16. | Draw Ellipse | | 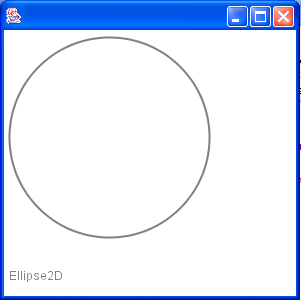 |
17. | Fill a Rectangle 2 | | 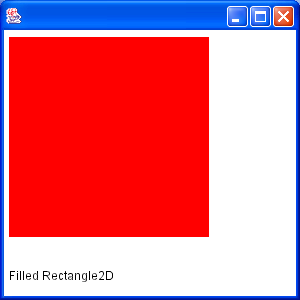 |
18. | Fill Arc 2 | | 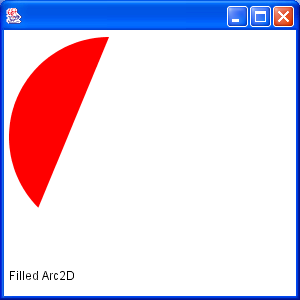 |
19. | Draw text | |  |
20. | Draw unicode string | | 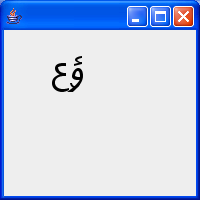 |
21. | Shape combine | | 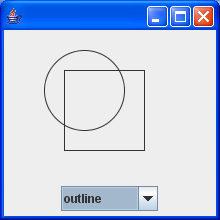 |
22. | Effects | | 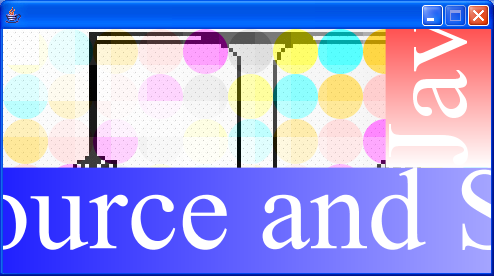 |
23. | Mouse drag and drop to draw | | 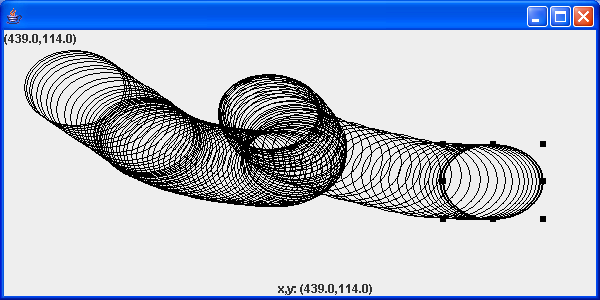 |
24. | Arc demonstration: scale, move, rotate, sheer | | 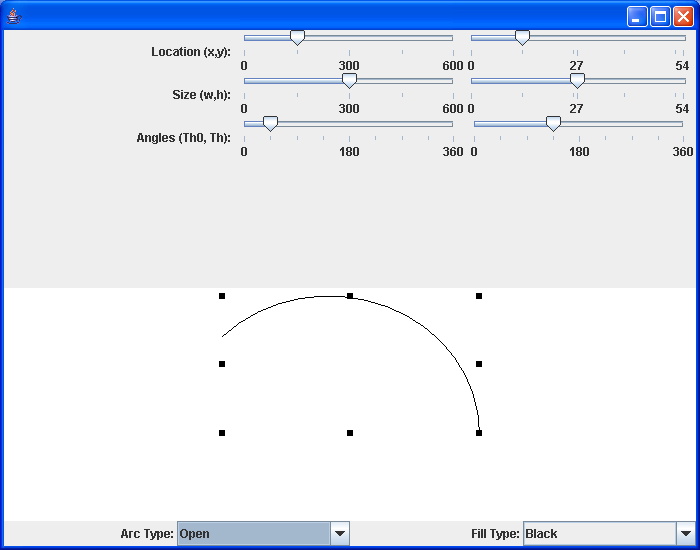 |
25. | Hypnosis Spiral | | 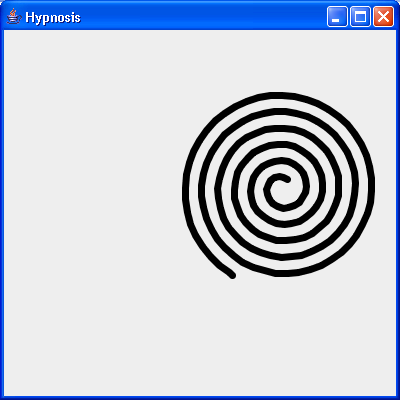 |
26. | GlyphVector.getNumGlyphs() | | |
27. | Resize a shape | | |
28. | Rectangle with rounded corners drawn using Java 2D Graphics API | | |
29. | Compares two ellipses and returns true if they are equal or both null. | | |
30. | Compares two lines are returns true if they are equal or both null. | | |
31. | Creates a diagonal cross shape. | | |
32. | Creates a diamond shape. | | |
33. | Creates a new Stroke-Object for the given type and with. | | |
34. | Creates a region surrounding a line segment by 'widening' the line segment. | | |
35. | Creates a triangle shape that points downwards. | | |
36. | Creates a triangle shape that points upwards. | | |
37. | Generate Polygon | | |
38. | Polygon with float coordinates. | | |
39. | Polyline 2D | | |
40. | Serialises a Shape object. | | |
41. | Tests two polygons for equality. If both are null this method returns true. | | |
42. | Union two rectangles | | |
43. | Calculate Intersection Clip | | |
44. | Draws a shape with the specified rotation about (x, y). | | |
45. | Checks, whether the given rectangle1 fully contains rectangle 2 (even if rectangle 2 has a height or width of zero!). | | |
46. | Reads a Point2D object that has been serialised by the writePoint2D(Point2D, ObjectOutputStream)} method. | | |
47. | Returns a point based on (x, y) but constrained to be within the bounds of a given rectangle. | | |
48. | RectListManager is a class to manage a list of rectangular regions. | | |
49. | This program demonstrates the various 2D shapes | | 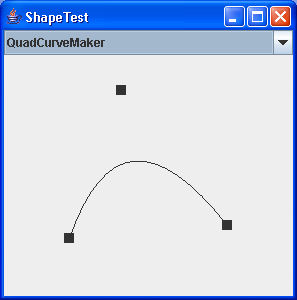 |