Calculate the arithmetic function
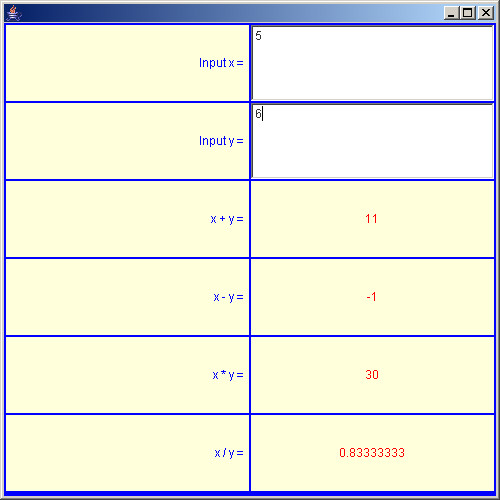
/*************************************************************************
* *
* This source code file, and compiled classes derived from it, can *
* be used and distributed without restriction, including for commercial *
* use. (Attribution is not required but is appreciated.) *
* *
* David J. Eck *
* Department of Mathematics and Computer Science *
* Hobart and William Smith Colleges *
* Geneva, New York 14456, USA *
* Email: eck@hws.edu WWW: http://math.hws.edu/eck/ *
* *
*************************************************************************/
import java.awt.*;
import java.applet.Applet;
import edu.hws.jcm.data.*; // Packages that define some JCM classes.
import edu.hws.jcm.awt.*;
public class ArithmeticApplet extends Applet {
/* The makeLabel() routine is just a small utility routine
that makes a label containing a given string. Labels made with
this routine are used in the little boxes on the left side
of the above applet. */
Label makeLabel(String str) {
Label lab = new Label(str, Label.RIGHT);
lab.setBackground(new Color(255,255,220));
lab.setForeground(Color.blue);
return lab;
}
/* The makeDisplayLable() routine is a small utility routine
that makes a "DisplayLabel". A DisplayLabel is one of the
JCM components whose purpose is to display one or more
Values. A "Value" is just an object that implements the
interface edu.hws.jcm.data.Value. It has an assoicated numeric
value that can be retrieved with getVal(). This is a fundamental
interface in the JCM system which can represent constant
values, variables, and results of computations.
DisplayLabels are used for the lower four boxes on the
right of the above applet. */
Label makeDisplayLabel(Value val) {
Label lab = new DisplayLabel("#",val);
// In the string displayed by this label, the # is
// replaced by the value of val.
lab.setBackground(new Color(255,255,220));
lab.setForeground(Color.red);
lab.setAlignment(Label.CENTER);
return lab;
}
/* The standard Applet routine, init(), sets up and lays out
the applet. For a JCM Applet, there is usually not much to
do besides setting it up. The JCM components are "active",
and they pretty much take care of themselves if they are
set up properly. */
public void init() {
setBackground(Color.blue); // Set the background color and
setLayout(new BorderLayout()); // layout of the applet as a whole.
// The whole applet will be filled with
// a single JCMPanel.
JCMPanel main = new JCMPanel(6,2,2); // The applet will be built with
// JCMPanels, a subclass of Panel,
// defined in edu.hws.jcm.awt.
// If an applet is constructed using
// JCMPanels, a lot of the JCM setup
// is taken care of automatically
// (at some possible loss of efficiency).
// This JCMPanel constructor creates
// a panel with a GridLayout with
// 6 rows and two columns, and with
// 2-pixel gaps between the rows
// and columns.
add(main, BorderLayout.CENTER); // The JCMPanel will fill the whole applet.
main.setInsetGap(2); // This sets the "Insets" around the edges of the panel.
// It allows a two-pixel-wide blue border to show
// around the edges.
Parser parser = new Parser(); // A Parser can be used to turn strings
// into Expressions. An Expression is a
// Value that is computed from some mathematical
// formula such as x+y or 3*sin(x^2-2).
VariableInput xInput = new VariableInput("x","0",parser);
VariableInput yInput = new VariableInput("y","0",parser);
// A VariableInput is a text input box where the user can
// input the value of a variable. The variable can be used
// in Expressions, provided that the variable is registered
// with the Parser that makes the Expressions. The two lines
// above make two VariableInputs for inputting the values of
// two variables. The names of the variables are "x" and
// "y", and they are registered with the Parser named parser
// which was created above.
main.add(makeLabel("Input x = ")); // Add components for the top row of the applet.
main.add(xInput);
main.add(makeLabel("Input y = ")); // Components for the second row.
main.add(yInput);
main.add(makeLabel("x + y = ")); // Components for the third row.
main.add(makeDisplayLabel( parser.parse("x+y") ));
// Here, parser.parse("x+y") creates an Expression that represents
// the value of the formula x+y. x and y are the names for the
// values in the input boxes xInput and yInput, so this formula
// represents the sum of the numbers input by the user. Note that
// and Expression is a type of Value object, and so can be passed
// to the makeDisplayLabel() routine, which is defined above.
// This routine creates a label that shows the value of the
// Expression.
// Note: For the simple expression x+y, I could have used the
// ValueMath class to create the value object, instead of a parser.
// Just replace parser.parse("x+y") with: new ValueMath(xInput,yInput,'+').
// ValueMath objects would let me do without the parser entirely in
// this applet, but parsers are necessary for more complicated expressions,
// and they are used for expressions entered by the user.
main.add(makeLabel("x - y = ")); // Components for the fourth row.
main.add(makeDisplayLabel( parser.parse("x-y") ));
main.add(makeLabel("x * y = ")); // Components for the fifth row.
main.add(makeDisplayLabel( parser.parse("x*y") ));
main.add(makeLabel("x / y = ")); // Components for the sixth row.
main.add(makeDisplayLabel( parser.parse("x/y") ));
Controller c = main.getController(); // A Controller is another of the
// fundamental JCM classes. A controller
// makes things happen. It "listens" for
// user actions and does the computations
// necessary to update any JCM components
// that it controls. A JCMPanel has a
// controller for recomputing the JCM
// components in the panel. Calling
// main.getController() retrieves the
// Controller for the JCMPanel, main.
xInput.setOnTextChange(c); // A Controller must still be told what user
yInput.setOnTextChange(c); // actions to listen for. These two lines set
// up the Controller, c, to listen for any
// changes in the text in the input boxes
// xInput and yInput. Thus, every time the
// user types a character in one of these
// boxes, all the DisplayLabels are recomputed.
} // end init()
public static void main(String[] a){
javax.swing.JFrame f = new javax.swing.JFrame();
java.applet.Applet app = new ArithmeticApplet();
app.init();
f.getContentPane().add (app);
f.pack();
f.setSize (new Dimension (500, 500));
f.setVisible(true);
}
} // end class ArithmeticApplet
jcm1-source.zip( 532 k)Related examples in the same category