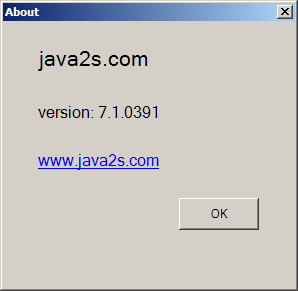
Imports System.Windows.Forms
public class AboutDialog
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents Button1 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Button1 = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'Button1
'
Me.Button1.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Button1.Location = New System.Drawing.Point(160, 168)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(80, 32)
Me.Button1.TabIndex = 0
Me.Button1.Text = "About"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 266)
Me.Controls.Add(Me.Button1)
Me.ResumeLayout(False)
End Sub
Private Sub showaboutBox()
Dim aboutbox As New About
aboutbox.ShowDialog(Me)
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
ShowAboutBox()
End Sub
End Class
Public Class About
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents LinkLabel1 As System.Windows.Forms.LinkLabel
Friend WithEvents Button1 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Label1 = New System.Windows.Forms.Label
Me.Label2 = New System.Windows.Forms.Label
Me.LinkLabel1 = New System.Windows.Forms.LinkLabel
Me.Button1 = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'Label1
'
Me.Label1.Font = New System.Drawing.Font("Microsoft Sans Serif", 15.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Label1.Location = New System.Drawing.Point(32, 24)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(152, 32)
Me.Label1.TabIndex = 0
Me.Label1.Text = "java2s.com"
'
'Label2
'
Me.Label2.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Label2.Location = New System.Drawing.Point(32, 80)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(144, 24)
Me.Label2.TabIndex = 1
Me.Label2.Text = "version: 7.1.0391"
'
'LinkLabel1
'
Me.LinkLabel1.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.LinkLabel1.Location = New System.Drawing.Point(32, 128)
Me.LinkLabel1.Name = "LinkLabel1"
Me.LinkLabel1.Size = New System.Drawing.Size(160, 32)
Me.LinkLabel1.TabIndex = 2
Me.LinkLabel1.TabStop = True
Me.LinkLabel1.Text = "www.java2s.com"
'
'Button1
'
Me.Button1.DialogResult = System.Windows.Forms.DialogResult.OK
Me.Button1.Location = New System.Drawing.Point(176, 176)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(80, 32)
Me.Button1.TabIndex = 3
Me.Button1.Text = "OK"
'
'About
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 266)
Me.Controls.Add(Me.Button1)
Me.Controls.Add(Me.LinkLabel1)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.Label1)
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.Name = "About"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterParent
Me.Text = "About"
Me.ResumeLayout(False)
End Sub
Private Sub LinkLabel1_LinkClicked(ByVal sender As System.Object, ByVal e As System.Windows.Forms.LinkLabelLinkClickedEventArgs) Handles LinkLabel1.LinkClicked
System.Diagnostics.Process.Start("http://www.java2s.com")
End Sub
End Class