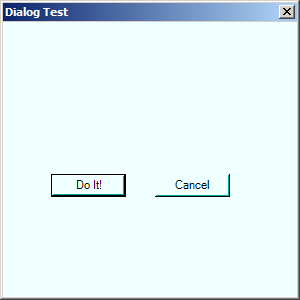
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Drawing.Drawing2D
public class CreateDialogBaseOnForm
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class DlgTest
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents btnOK As System.Windows.Forms.Button
Friend WithEvents btnCancel As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnOK = New System.Windows.Forms.Button()
Me.btnCancel = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'btnOK
'
Me.btnOK.DialogResult = System.Windows.Forms.DialogResult.OK
Me.btnOK.Location = New System.Drawing.Point(48, 152)
Me.btnOK.Name = "btnOK"
Me.btnOK.TabIndex = 0
Me.btnOK.Text = "Do It!"
'
'btnCancel
'
Me.btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel
Me.btnCancel.Location = New System.Drawing.Point(152, 152)
Me.btnCancel.Name = "btnCancel"
Me.btnCancel.TabIndex = 1
Me.btnCancel.Text = "Cancel"
'
'DlgTest
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.btnCancel, Me.btnOK})
Me.Name = "DlgTest"
Me.Text = "dlg"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btnOK_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOK.Click
DialogResult = DialogResult.OK
End Sub
Private Sub btnCancel_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCancel.Click
DialogResult = DialogResult.Cancel
End Sub
End Class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents btnCreate As System.Windows.Forms.Button
Friend WithEvents lblReturn As System.Windows.Forms.Label
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnCreate = New System.Windows.Forms.Button()
Me.lblReturn = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'btnCreate
'
Me.btnCreate.Location = New System.Drawing.Point(96, 152)
Me.btnCreate.Name = "btnCreate"
Me.btnCreate.Size = New System.Drawing.Size(120, 23)
Me.btnCreate.TabIndex = 0
Me.btnCreate.Text = "Create Dialog Box"
'
'lblReturn
'
Me.lblReturn.Location = New System.Drawing.Point(96, 96)
Me.lblReturn.Name = "lblReturn"
Me.lblReturn.TabIndex = 1
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblReturn, Me.btnCreate})
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btnCreate_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnCreate.Click
dim dlg as New DlgTest
dlg.Text = "Dialog Test"
dlg.FormBorderStyle = FormBorderStyle.FixedDialog
dlg.BackColor = Color.Azure
dlg.ControlBox = true
dlg.MaximizeBox = false
dlg.MinimizeBox = false
dlg.ShowInTaskbar = false
'dlg.Icon = new Icon(f.GetType(),"YourFile.ICO")
dlg.Size = new Size(300,300)
dlg.StartPosition = FormStartPosition.CenterScreen
select case dlg.ShowDialog()
case DialogResult.Abort
lblReturn.Text = "Abort, Abort"
case DialogResult.Cancel
lblReturn.Text = "You have cancelled."
case DialogResult.OK
lblReturn.Text = "I'm OK, You're OK"
case else
lblReturn.Text = "Whatever..."
end select
End Sub
End Class