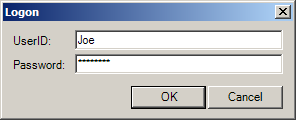
Imports System.Windows.Forms
public class LoginDialog
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
components = New System.ComponentModel.Container()
Me.Text = "Form1"
End Sub
#End Region
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim frmLogon As New LogonDialog()
Dim success As Boolean = False
Dim errorMessage As String
If frmLogon.ShowDialog() = DialogResult.OK Then
If frmLogon.txtUserid.Text = "Joe" Then
If frmLogon.txtPassword.Text = "password" Then
success = True
Else
errorMessage = "Invalid Password!"
End If
Else
errorMessage = "Invalid User ID!"
End If
Else
errorMessage = "Logon Attempt Cancelled"
End If
If Not success Then
MessageBox.Show(errorMessage, "Logon Failed", MessageBoxButtons.OK, MessageBoxIcon.Error)
Me.Close()
End If
End Sub
End Class
Public Class LogonDialog
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents btnOK As System.Windows.Forms.Button
Friend WithEvents btnCancel As System.Windows.Forms.Button
Friend WithEvents lblUserid As System.Windows.Forms.Label
Friend WithEvents lblPassword As System.Windows.Forms.Label
Friend WithEvents txtUserid As System.Windows.Forms.TextBox
Friend WithEvents txtPassword As System.Windows.Forms.TextBox
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnOK = New System.Windows.Forms.Button()
Me.btnCancel = New System.Windows.Forms.Button()
Me.txtUserid = New System.Windows.Forms.TextBox()
Me.txtPassword = New System.Windows.Forms.TextBox()
Me.lblUserid = New System.Windows.Forms.Label()
Me.lblPassword = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'btnOK
'
Me.btnOK.Location = New System.Drawing.Point(128, 64)
Me.btnOK.Name = "btnOK"
Me.btnOK.TabIndex = 0
Me.btnOK.Text = "OK"
'
'btnCancel
'
Me.btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel
Me.btnCancel.Location = New System.Drawing.Point(205, 64)
Me.btnCancel.Name = "btnCancel"
Me.btnCancel.TabIndex = 1
Me.btnCancel.Text = "Cancel"
'
'txtUserid
'
Me.txtUserid.Location = New System.Drawing.Point(72, 8)
Me.txtUserid.Name = "txtUserid"
Me.txtUserid.Size = New System.Drawing.Size(208, 20)
Me.txtUserid.TabIndex = 2
Me.txtUserid.Text = "Joe"
'
'txtPassword
'
Me.txtPassword.Location = New System.Drawing.Point(72, 32)
Me.txtPassword.Name = "txtPassword"
Me.txtPassword.PasswordChar = ChrW(42)
Me.txtPassword.Size = New System.Drawing.Size(208, 20)
Me.txtPassword.TabIndex = 3
Me.txtPassword.Text = "password"
'
'lblUserid
'
Me.lblUserid.AutoSize = True
Me.lblUserid.Location = New System.Drawing.Point(8, 12)
Me.lblUserid.Name = "lblUserid"
Me.lblUserid.Size = New System.Drawing.Size(43, 13)
Me.lblUserid.TabIndex = 4
Me.lblUserid.Text = "UserID:"
'
'lblPassword
'
Me.lblPassword.AutoSize = True
Me.lblPassword.Location = New System.Drawing.Point(8, 36)
Me.lblPassword.Name = "lblPassword"
Me.lblPassword.Size = New System.Drawing.Size(57, 13)
Me.lblPassword.TabIndex = 5
Me.lblPassword.Text = "Password:"
'
'LogonDialog
'
Me.AcceptButton = Me.btnOK
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.CancelButton = Me.btnCancel
Me.ClientSize = New System.Drawing.Size(290, 95)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblPassword, Me.lblUserid, Me.txtPassword, Me.txtUserid, Me.btnCancel, Me.btnOK})
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.Name = "LogonDialog"
Me.Text = "Logon"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btnOK_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOK.Click
Dim sUserID As String
Dim sPassword As String
sUserID = txtUserid.Text
sPassword = txtPassword.Text
If sUserID.Trim() = "" Then
MessageBox.Show("UserID cannot be blank.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
txtUserid.Select()
Else
If sPassword.Trim() = "" Then
MessageBox.Show("Password cannot be blank.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
txtPassword.Select()
Else
Me.DialogResult = DialogResult.OK
End If
End If
End Sub
End Class