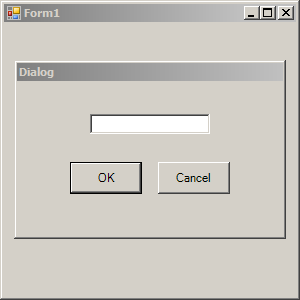
Imports System.Windows.Forms
public class UseDefineDialog
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If Disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(Disposing)
End Sub
Private WithEvents btnNewCaption As System.Windows.Forms.Button
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnNewCaption = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'btnNewCaption
'
Me.btnNewCaption.Location = New System.Drawing.Point(106, 120)
Me.btnNewCaption.Name = "btnNewCaption"
Me.btnNewCaption.Size = New System.Drawing.Size(80, 32)
Me.btnNewCaption.TabIndex = 0
Me.btnNewCaption.Text = "New Caption"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.btnNewCaption})
Me.Name = "Form1"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btnNewCaption_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnNewCaption.Click
Dim frmCaption As New Form2()
frmCaption.ShowDialog()
If frmCaption.DialogResult = DialogResult.OK Then
Me.Text = frmCaption.Caption
End If
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
End Sub
End Class
Public Class Form2
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If Disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(Disposing)
End Sub
Private WithEvents btnOK As System.Windows.Forms.Button
Private WithEvents btnCancel As System.Windows.Forms.Button
Private WithEvents txtNewCaption As System.Windows.Forms.TextBox
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThroughAttribute()> Private Sub InitializeComponent()
Me.btnCancel = New System.Windows.Forms.Button()
Me.txtNewCaption = New System.Windows.Forms.TextBox()
Me.btnOK = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'btnCancel
'
Me.btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel
Me.btnCancel.Location = New System.Drawing.Point(141, 80)
Me.btnCancel.Name = "btnCancel"
Me.btnCancel.Size = New System.Drawing.Size(72, 32)
Me.btnCancel.TabIndex = 2
Me.btnCancel.Text = "Cancel"
'
'txtNewCaption
'
Me.txtNewCaption.Location = New System.Drawing.Point(73, 32)
Me.txtNewCaption.Name = "txtNewCaption"
Me.txtNewCaption.Size = New System.Drawing.Size(120, 20)
Me.txtNewCaption.TabIndex = 1
Me.txtNewCaption.Text = ""
'
'btnOK
'
Me.btnOK.DialogResult = System.Windows.Forms.DialogResult.OK
Me.btnOK.Location = New System.Drawing.Point(53, 80)
Me.btnOK.Name = "btnOK"
Me.btnOK.Size = New System.Drawing.Size(72, 32)
Me.btnOK.TabIndex = 0
Me.btnOK.Text = "OK"
'
'Form2
'
Me.AcceptButton = Me.btnOK
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.CancelButton = Me.btnCancel
Me.ClientSize = New System.Drawing.Size(266, 154)
Me.ControlBox = False
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.btnCancel, Me.txtNewCaption, Me.btnOK})
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog
Me.Name = "Form2"
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterParent
Me.Text = "Dialog"
Me.ResumeLayout(False)
End Sub
#End Region
Public Property Caption() As String
Get
Caption = txtNewCaption.Text
End Get
Set(ByVal Value As String)
End Set
End Property
End Class