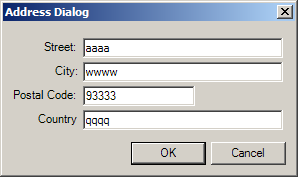
Imports System.Windows.Forms
public class DialogWithProperties
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents Button1 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Button1 = New System.Windows.Forms.Button
Me.SuspendLayout()
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(104, 88)
Me.Button1.Name = "Button1"
Me.Button1.TabIndex = 0
Me.Button1.Text = "Button1"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 266)
Me.Controls.Add(Me.Button1)
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub Button1_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles Button1.Click
Dim address As New addressDialog
Dim Street As String = "aaaa"
Dim City As String = "wwww"
Dim Country As String = "qqqq"
Dim PostalCode As String = "93333"
address.Street = Street
address.City = City
address.Country = Country
address.PostalCode = PostalCode
If address.ShowDialog = DialogResult.OK Then
Street = address.Street
City = address.City
Country = address.Country
PostalCode = address.PostalCode
End If
End Sub
End Class
Public Class addressDialog
Inherits System.Windows.Forms.Form
Public Property Street() As String
Get
Return Me.txtStreet.Text
End Get
Set(ByVal Value As String)
Me.txtStreet.Text = Value
End Set
End Property
Public Property City() As String
Get
Return Me.txtCity.Text
End Get
Set(ByVal Value As String)
Me.txtCity.Text = Value
End Set
End Property
Public Property PostalCode() As String
Get
Return Me.txtPostalCode.Text
End Get
Set(ByVal Value As String)
Me.txtPostalCode.Text = Value
End Set
End Property
Public Property Country() As String
Get
Return Me.txtCountry.Text
End Get
Set(ByVal Value As String)
Me.txtCountry.Text = Value
End Set
End Property
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents saveAddress As System.Windows.Forms.Button
Friend WithEvents cancelAddress As System.Windows.Forms.Button
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents Label3 As System.Windows.Forms.Label
Friend WithEvents Label4 As System.Windows.Forms.Label
Friend WithEvents txtStreet As System.Windows.Forms.TextBox
Friend WithEvents txtCity As System.Windows.Forms.TextBox
Friend WithEvents txtPostalCode As System.Windows.Forms.TextBox
Friend WithEvents txtCountry As System.Windows.Forms.TextBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.saveAddress = New System.Windows.Forms.Button
Me.cancelAddress = New System.Windows.Forms.Button
Me.txtStreet = New System.Windows.Forms.TextBox
Me.txtCity = New System.Windows.Forms.TextBox
Me.txtPostalCode = New System.Windows.Forms.TextBox
Me.txtCountry = New System.Windows.Forms.TextBox
Me.Label1 = New System.Windows.Forms.Label
Me.Label2 = New System.Windows.Forms.Label
Me.Label3 = New System.Windows.Forms.Label
Me.Label4 = New System.Windows.Forms.Label
Me.SuspendLayout()
'
'saveAddress
'
Me.saveAddress.Anchor = CType((System.Windows.Forms.AnchorStyles.Bottom Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.saveAddress.DialogResult = System.Windows.Forms.DialogResult.OK
Me.saveAddress.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.saveAddress.Location = New System.Drawing.Point(128, 120)
Me.saveAddress.Name = "saveAddress"
Me.saveAddress.TabIndex = 0
Me.saveAddress.Text = "OK"
'
'cancelAddress
'
Me.cancelAddress.Anchor = CType((System.Windows.Forms.AnchorStyles.Bottom Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.cancelAddress.DialogResult = System.Windows.Forms.DialogResult.Cancel
Me.cancelAddress.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.cancelAddress.Location = New System.Drawing.Point(208, 120)
Me.cancelAddress.Name = "cancelAddress"
Me.cancelAddress.TabIndex = 1
Me.cancelAddress.Text = "Cancel"
'
'txtStreet
'
Me.txtStreet.Location = New System.Drawing.Point(80, 16)
Me.txtStreet.Name = "txtStreet"
Me.txtStreet.Size = New System.Drawing.Size(200, 20)
Me.txtStreet.TabIndex = 2
Me.txtStreet.Text = "txtStreet"
'
'txtCity
'
Me.txtCity.Location = New System.Drawing.Point(80, 40)
Me.txtCity.Name = "txtCity"
Me.txtCity.Size = New System.Drawing.Size(200, 20)
Me.txtCity.TabIndex = 3
Me.txtCity.Text = "txtCity"
'
'txtPostalCode
'
Me.txtPostalCode.Location = New System.Drawing.Point(80, 64)
Me.txtPostalCode.Name = "txtPostalCode"
Me.txtPostalCode.Size = New System.Drawing.Size(112, 20)
Me.txtPostalCode.TabIndex = 4
Me.txtPostalCode.Text = "txtPostalCode"
'
'txtCountry
'
Me.txtCountry.Location = New System.Drawing.Point(80, 88)
Me.txtCountry.Name = "txtCountry"
Me.txtCountry.Size = New System.Drawing.Size(200, 20)
Me.txtCountry.TabIndex = 5
Me.txtCountry.Text = "txtCountry"
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(39, 18)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(38, 16)
Me.Label1.TabIndex = 6
Me.Label1.Text = "Street:"
'
'Label2
'
Me.Label2.AutoSize = True
Me.Label2.Location = New System.Drawing.Point(50, 42)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(27, 16)
Me.Label2.TabIndex = 7
Me.Label2.Text = "City:"
'
'Label3
'
Me.Label3.AutoSize = True
Me.Label3.Location = New System.Drawing.Point(8, 66)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(69, 16)
Me.Label3.TabIndex = 8
Me.Label3.Text = "Postal Code:"
'
'Label4
'
Me.Label4.AutoSize = True
Me.Label4.Location = New System.Drawing.Point(33, 90)
Me.Label4.Name = "Label4"
Me.Label4.Size = New System.Drawing.Size(44, 16)
Me.Label4.TabIndex = 9
Me.Label4.Text = "Country"
'
'addressDialog
'
Me.AcceptButton = Me.saveAddress
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.CancelButton = Me.cancelAddress
Me.ClientSize = New System.Drawing.Size(292, 152)
Me.Controls.Add(Me.Label4)
Me.Controls.Add(Me.Label3)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.Label1)
Me.Controls.Add(Me.txtCountry)
Me.Controls.Add(Me.txtPostalCode)
Me.Controls.Add(Me.txtCity)
Me.Controls.Add(Me.txtStreet)
Me.Controls.Add(Me.cancelAddress)
Me.Controls.Add(Me.saveAddress)
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.Name = "addressDialog"
Me.Text = "Address Dialog"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub saveAddress_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles saveAddress.Click
Me.Close()
End Sub
Private Sub cancelAddress_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cancelAddress.Click
Me.Close()
End Sub
End Class